Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial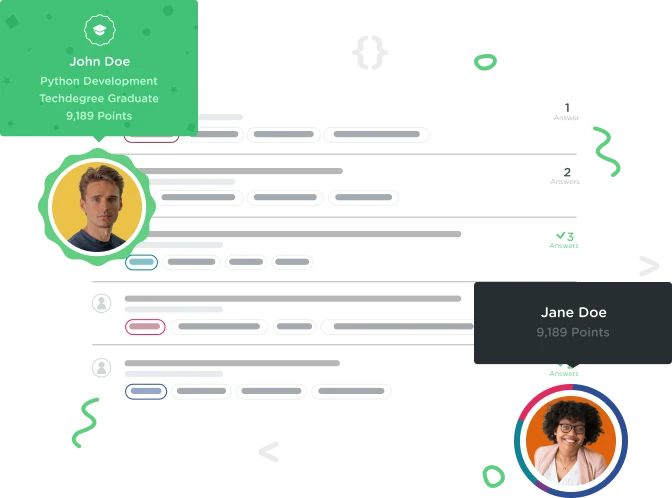
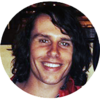
Michael Paccione
9,017 PointsDiv collision detection with viewport edges
Hi, I am building a game for an assignment and I need the player div(.circle) to stay within the viewport. When it hits a viewport edge it needs to bounce off.
I have this coded just about right but my div circle is not returning the right position values. It's possible that the function is getting the position before the animation is complete despite me coding the jQuery animation function to call the getCirclePos after animation is complete. I think this is it but even if it does register a viewport edge hit then I get essentially a function execute loop - I think from the if statements. I'm kind of stuck here :/
Here is all the code - have a look please :)
$(document).ready(function(){
//Cache Vars
var instructions = $('.instructions');
//Object Vars for Collision Detection
var screenLeft = {
x: 0,
y: window.innerHeight
};
var screenTop = {
x: window.innerWidth,
y: 0
};
var screenRight = {
x: window.innerWidth,
y: window.innerHeight
};
var screenBottom = {
x: window.innerWidth,
y: window.innerHeight
};
//Functions
//Move then get position values
function animateCircle(x, y){
$('.circle').animate({top: '+='+y, left: '+='+x},{ complete: getCirclePos()});
};
//Get circle position
function getCirclePos(){
var circleBound = $('.circle').offset();//document.getElementsByClassName('circle')[0].getBoundingClientRect();
var circlePos = {};
circlePos.width = $('.circle').width();
circlePos.height = $('.circle').height();
circlePos.left = circleBound.left;
circlePos.top = circleBound.top;
circlePos.right = circleBound.left+circlePos.width;
circlePos.bottom = circleBound.top+circlePos.height;
//Check for Screen Collision
console.log("circlePosLeft: "+circlePos.left+" screenLeft: "+screenLeft.x);
console.log("circlePosRight: "+circlePos.right+" screenRight: "+screenRight.x);
console.log("circlePosTop: "+circlePos.top+" screenTop: "+screenTop.y);
console.log("circlePosBottom: "+circlePos.bottom+" screenBottom: "+screenBottom.y);
if (circlePos.left <= 0){
animateCircle(-displaceX, 0);
console.log("Bounce off left");
}
if (circlePos.top <= 0){
animateCircle(0, -displaceY);
console.log("Bounce off top");
}
if (circlePos.right >= screenRight.x) {
animateCircle(-displaceX, 0);
console.log("Bounce off right");
}
if (circlePos.bottom >= screenBottom.y) {
animateCircle(0, -displaceY);
console.log("Bounce off bottom");
}
};
$('.circle').one('mouseenter', function(){
instructions.fadeTo("fast", 0);
setTimeout(function(){
instructions.hide()
}, 1000);
});
$('.circle').on("mouseenter", function circleMove(){
//Create XY Displacement Values
window.displaceX = Math.floor(Math.random() * (499 - 1)) + 1;
window.displaceY = Math.floor(Math.random() * (499 - 1)) + 1;
//50% Chance to move left/down
if (displaceX < 300){
displaceX *= -1;
}
if (displaceY < 300){
displaceY *= -1;
}
animateCircle(displaceX, displaceY);
});
});
html, body {
margin: 0 auto;
padding: 0;
height: 100%;
width: 100%;
overflow: hidden;
background-color: #000;
}
.circle {
border-radius: 50%;
height: 100px;
width: 100px;
background-color: #fff;
position: absolute;
}
.centerPos, .instructions {
top: 50%;
left: 50%;
transform: translateX(-50%) translateY(-50%);
-webkit-transform: translateX(-50%) translateY(-50%);
-moz-transform: translateX(-50%) translateY(-50%);
-ms-transform: translateX(-50%) translateY(-50%);
-o-transform: translateX(-50%) translateY(-50%);
/* transition: all 1s ease-in-out;
-webkit-transition: all 1s ease-in-out;*/
}
.instructions {
position: absolute;
width: 150px;
height: 50px;
top: 60%;
left: 51.3%;
color: #fcfcfc;
}
Michael Paccione
9,017 PointsMichael Paccione
9,017 PointsIn case you want to run it to debug