Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial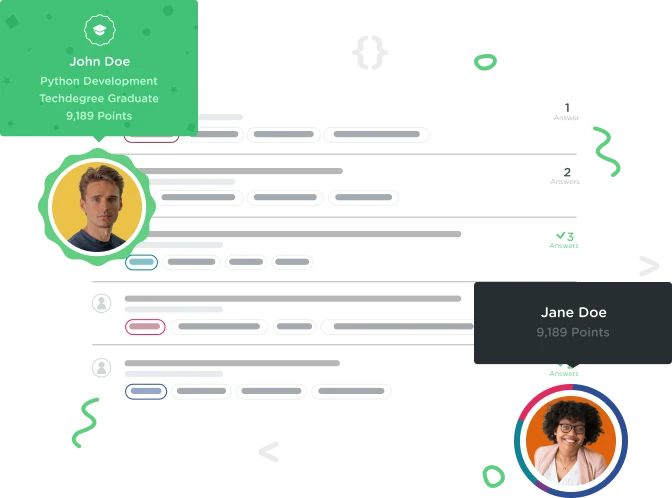

Y B
14,136 PointsDjango 1st unittest challenge
There is something that I'm not doing quite right for this unittest but I'm not sure what?
from django.test import TestCase
from .models import Writer
class WriterModelTestCase(TestCase):
'''Tests for the Writer model'''
def writer_creation(self):
writer = Writer.create(
name= 'Bob'
email = 'bob@moo.com'
bio = 'blah de blah'
)
self.assertIn(email, writer.mailto())
from django.db import models
class Article(models.Model):
headline = models.CharField(max_length=255)
publish_date = models.DateTimeField()
content = models.TextField()
writer = models.ForeignKey('Writer')
def __str__(self):
return self.headline
class Writer(models.Model):
name = models.CharField(max_length=255)
email = models.EmailField()
bio = models.TextField()
def __str__(self):
return self.name
def mailto(self):
return '{} <{}>'.format(self.name, self.email)
4 Answers

Ken Alger
Treehouse TeacherY B;
When testing, our test names have to follow a certain format. If you re-watch the video, or recall from the Python Testing course, test names need to start with "test". That should take care of your code to get through the challenge.
Happy coding,
Ken
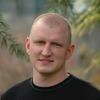
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsY B, I'm not sure if you got this working yet or not.
The generic error you keep getting should be telling you to check your Functions name. Like Ken Alger said, a functions Test Name must follow a certain naming format to get this to pass. I also spent more time than necessary picking apart why my code was failing because the error was too generic. Everything in my code was fine except the name of the function.

Y B
14,136 PointsGo it working thanks Ken's answer fixed it.
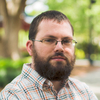
Kenneth Love
Treehouse Guest TeacherThe prompt says to test that the email
attribute is in the mailto()
method. You're testing for email
which isn't defined.

Y B
14,136 PointsThanks I fixed another couple of silly errors, but still can't get it to work unfortunately. Hard to debug as the challenge just says your test didn't pass. If I put it in a separate editor for some reason the test doesn't run?
from django.test import TestCase
from .models import Writer
class WriterModelTestCase(TestCase):
'''Tests for the Writer model'''
def writer_creation(self):
writer = Writer.objects.create(
name= 'Bob',
email = 'bob@moo.com',
bio = 'blah de blah',
)
self.assertIn(writer.email, writer.mailto())
Y B
14,136 PointsY B
14,136 PointsThanks- i didn't realise that the name of the test function name was checked. I take that is a requirement in the unites module?