Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial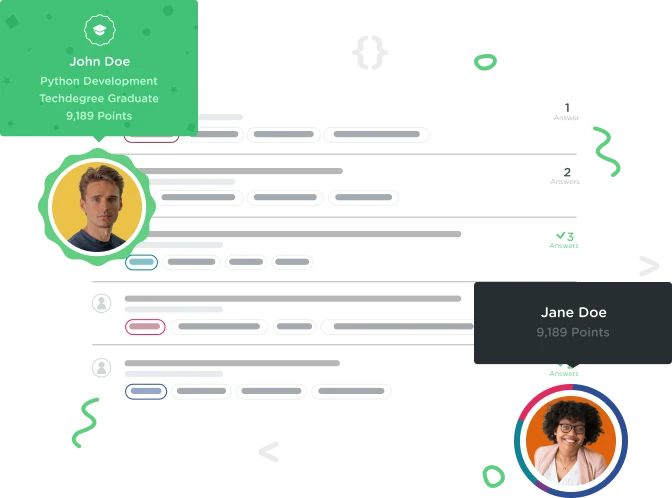
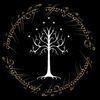
jacinator
11,936 PointsDjango annotation doesn't make sense
I've been stuck on this for a little while now, but I can't figure out Django annotation. The error that I keep getting is "Didn't find the right average." If someone can tell me what I am doing wrong and why it is wrong, I would greatly appreciate it.
https://teamtreehouse.com/library/django-orm/total-control/annotate is the code challenge that this is from.
import datetime
from django.db.models import Q, Avg
from django.shortcuts import render, get_object_or_404
from . import models
def good_reviews(request):
reviews = models.Review.objects.filter(rating__gte=3)
return render(request, 'products/reviews.html', {'reviews': reviews})
def recent_reviews(request):
six_months_ago = datetime.datetime.today() - datetime.timedelta(days=180)
reviews = models.Review.objects.exclude(created_at__lt=six_months_ago)
return render(request, 'products/reviews.html', {'reviews': reviews})
def product_detail(request, pk):
product = get_object_or_404(models.Product, pk=pk)
four_weeks_ago = datetime.datetime.today() - datetime.timedelta(weeks=4)
reviews = product.review_set.filter(
Q(rating__gte=8)|Q(created_at__gte=four_weeks_ago)
).order_by('-created_at').all()
return render(request, 'products/product_detail.html', {'product': product, 'reviews': reviews})
def product_list(request):
# This is my code here. Prior it was
# products = models.Product.objects.all()
products = models.Product.objects.all().annotate(
avg_rating=Avg('review', distinct=True)
)
return render(request, 'products/product_list.html', {'products': products})
1 Answer
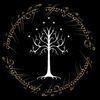
jacinator
11,936 PointsI needed to get the Avg
on review.rating
, not review
.
def product_list(request):
products = models.Product.objects.all().annotate(
avg_rating=Avg('review__rating', distinct=True)
)
return render(request, 'products/product_list.html', {'products': products})