Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial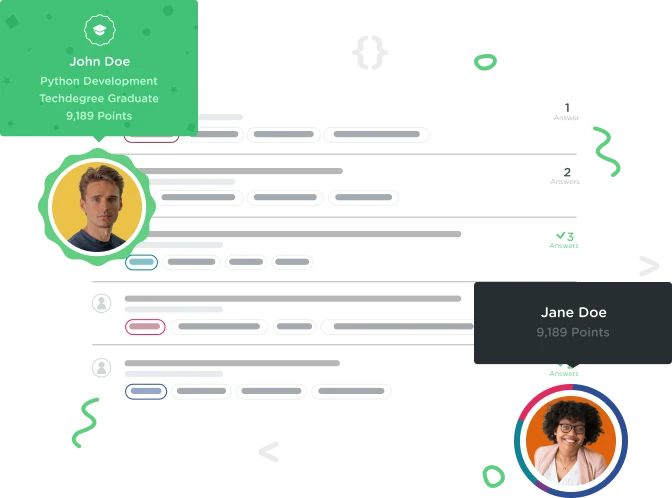

Y B
14,136 PointsDjango CBV custom queryset
Hmm this is hard. Not sure why this doesn't pass the challenge.
from django.views import generic
from . import models
class ArticleList(generic.ListView):
model = models.Article
class ArticleDetail(generic.DetailView):
model = models.Article
class ArticleCreate(generic.CreateView):
fields = ('title', 'body', 'author', 'published')
model = models.Article
class ArticleUpdate(generic.UpdateView):
fields = ('title', 'body', 'author', 'published')
model = models.Article
class ArticleDelete(generic.DeleteView):
model = models.Article
class ArticleSearch(generic.ListView):
model = models.Article
def get_queryset(self):
if self.kwargs['term']:
return self.model.objects.filter(body_icontains=self.kwargs['term'])
return EmptyQuerySet
3 Answers

Tatiana Vasilevskaya
Python Web Development Techdegree Graduate 28,600 PointsIf the term is blank, you need to return self.model.objects.none()
, which is an instance of an EmptyQuerySet
(none() docs)
Also, for field lookups you need two underscores body__icontains=self.kwargs['term']

Tatiana Vasilevskaya
Python Web Development Techdegree Graduate 28,600 PointsThere are some issues with your code. You are supposed to search for articles with a certain term in their body, however you are searching for articles, which body is equal to the lowercased term. You may want to consider using icontains for this.
Your get_queryset
method always returns None. Actually, it can't return None at all. Check the docs
Also, it should be self.model
in the method, not just model

Y B
14,136 PointsThanks, I've updated my code above. But I'm unsure whether I should return an EmptyQuerySet or just an empty list. Unfortunately I couldn't get either to work?

leakedmemory
10,868 Points@ Y B did you solve the your issue?
I just got it to pass.
def get_queryset(self):
term = self.kwargs['term']:
if not term:
return self.model.objects.none()
else:
return self.model.objects.filter(body__icontains=term)
As noted above, use two underscores between body and icontains.
Good Luck!