Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial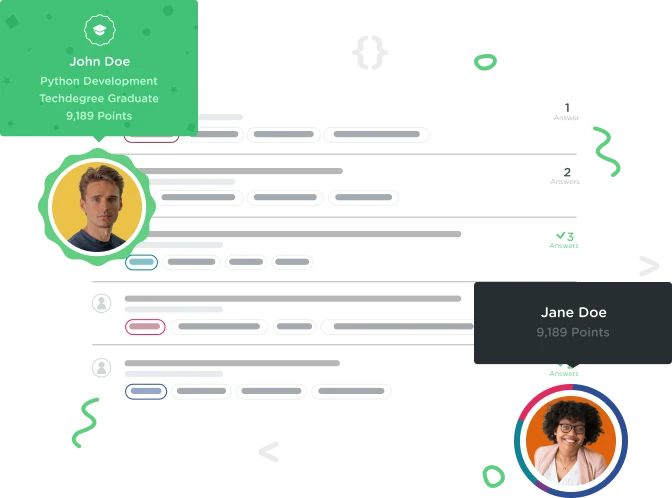
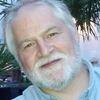
Jeff Muday
Treehouse Moderator 28,717 PointsDjango: display ImageField() with admin.site.register(model.MyModel) trick?
If I declare an ImageField() to be part of a model, and then subsequently register that model in the admin site, is there a way to display that ImageField() as a thumbnail?
For example, if I have a UserProfile picture, I would like to see it displayed as a picture/thumbnail in the admin interface rather than just the filename (as it currently is).
Is there a trick I am missing about getting an ImageField() to display in the interface, or is this not built into the current version of Django (1.9.x)?
1 Answer
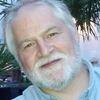
Jeff Muday
Treehouse Moderator 28,717 PointsI solved my issue after re-reading the Django project documentation on static files and admin site. This is probably not the most elegant solution, but it is working and can certainly use a few additional tweaks. I created a demo project and application as "proof of concept". In the sample "app" I have an image model. It works in the development environment, but haven't tested on a server environment, which may require a few additional tweaks.
from the sampleapp
models.py
import os
from django.db import models
from django.conf import settings
from django.utils.safestring import mark_safe
# the settings for MEDIA_ROOT and MEDIA_URL come from the project settings
# but could be overridden in the model
# MEDIA_ROOT = '/home/<user>/project/imgproject/media_cdn'
# MEDIA_URL = '/media'
# Create your models here.
class Image(models.Model):
# allows for an image to be either stored in the MEDIA_ROOT path or
# be a reference to an external URL to an image.
created_at = models.DateTimeField(auto_now_add=True)
title = models.CharField(max_length = 255)
description = models.TextField(blank=True)
image = models.ImageField(upload_to=settings.MEDIA_ROOT, blank=True)
externalURL = models.URLField(blank=True)
def url(self):
# returns a URL for either internal stored or external image url
if self.externalURL:
return self.externalURL
else:
# is this the best way to do this??
return os.path.join('/',settings.MEDIA_URL, os.path.basename(str(self.image)))
def image_tag(self):
# used in the admin site model as a "thumbnail"
return mark_safe('<img src="{}" width="150" height="150" />'.format(self.url()) )
image_tag.short_description = 'Image'
def __unicode__(self):
# add __str__() if using Python 3.x
return self.title
from sampleapp
admin.py
from django.contrib import admin
from .models import Image
class ImageAdmin(admin.ModelAdmin):
# explicitly reference fields to be shown, note image_tag is read-only
fields = ( 'image_tag','title','description','image','externalURL', )
readonly_fields = ('image_tag',)
admin.site.register(Image, ImageAdmin)