Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial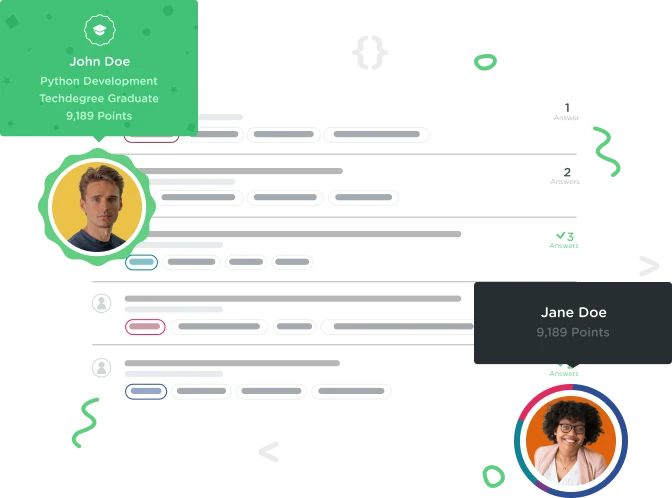

Jassim Alhatem
20,883 PointsDjango foreign keys. Website problem.
So I'm making a simple "Make your workout" website for fun. But currently, I'm getting stuck a little problem.
models.py
from django.db import models
from django.urls import reverse
DAYS_OF_WEEK = (
(0, 'Monday'),
(1, 'Tuesday'),
(2, 'Wednesday'),
(3, 'Thursday'),
(4, 'Friday'),
(5, 'Saturday'),
(6, 'Sunday'),
)
class Plan(models.Model):
title = models.CharField(max_length=100)
description = models.TextField(blank=True)
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
def get_absolute_url(self):
return reverse('workout:main')
DEFAULT_PLAN_ID = 1
class Workout(models.Model):
title = models.CharField(max_length=100, default="My Workout")
notes = models.TextField(blank=True)
created_at = models.DateTimeField(auto_now_add=True)
plan = models.ForeignKey(Plan, on_delete=models.CASCADE, related_name="workouts", default=DEFAULT_PLAN_ID)
day = models.CharField(choices=DAYS_OF_WEEK, max_length=1)
rest_day = models.BooleanField(default=False)
def __str__(self):
return self.title
class Exercise(models.Model):
Done = models.BooleanField(default=False)
exercise = models.CharField(max_length=100, default="")
sets = models.IntegerField(default=0)
reps = models.IntegerField(default=0)
workout = models.ForeignKey(Workout, related_name="exercises", on_delete=models.CASCADE)
plan = models.ForeignKey(Plan, related_name="exercises", on_delete=models.CASCADE, default=DEFAULT_PLAN_ID)
def __str__(self):
return self.exercise
So I have made cards (Which are the plans. For example, Push-Pull-Legs). And when the user clicks on one of them it takes him to a page where it shows him his workouts ( for that plan ). But there's a problem, I don't know how to show the user his workouts for that plan only.
views.py
from django.views.generic import CreateView, TemplateView, ListView
from django.contrib.auth.mixins import LoginRequiredMixin
from django.contrib import messages
from . import forms
from . import models
class MainPageView(TemplateView):
template_name = "main_page.html"
class AboutPageView(TemplateView):
template_name = "about.html"
class PlanListView(LoginRequiredMixin, ListView):
model = models.Plan
template_name = "my_workouts/my_plans.html"
def handle_no_permission(self):
messages.error(self.request, "You must be logged in!")
return super(PlanListView, self).handle_no_permission()
class WorkoutListView(LoginRequiredMixin, ListView):
model = models.Workout
template_name = "my_workouts/my_workouts.html"
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['workout_list'] = models.Workout.objects.all()
return context
class PlanForm(LoginRequiredMixin, CreateView):
model = models.Plan
form_class = forms.MakeWorkoutForm
template_name = "workouts/make_workout_form.html"
def handle_no_permission(self):
messages.error(self.request, "You must be logged in!")
return super(PlanForm, self).handle_no_permission()
urls.py
from django.urls import path
from . import views
app_name = 'workout'
urlpatterns = [
path('main', views.MainPageView.as_view(), name="main"),
path('about', views.AboutPageView.as_view(), name="about"),
path('make_workout', views.PlanForm.as_view(), name="make_workout_form"),
path('my_plans', views.PlanListView.as_view(), name="my_plans"),
path('my_workout/<int:pk>/', views.WorkoutListView.as_view(), name="my_workouts"),
]
{% extends "layout.html" %}
{% load static %}
{% block styles %} <link rel="stylesheet" href="{% static 'css/main-page.css' %}"> {% endblock %}
{% block title %} Plans {% endblock %}
{% block content %}
<div id="welcome-msg">
{% for item in object_list %}
<div class="card">
<h2 class="plan-title"><a href="{% url 'workout:my_workouts' item.pk %}">{{ item.title }}</a></h2>
<div class="in-card">
<p>{{ item.description }}</p>
</div>
</div>
{% endfor %}
</div>
{% endblock %}
Any help would be appreciated. Thanks!