Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial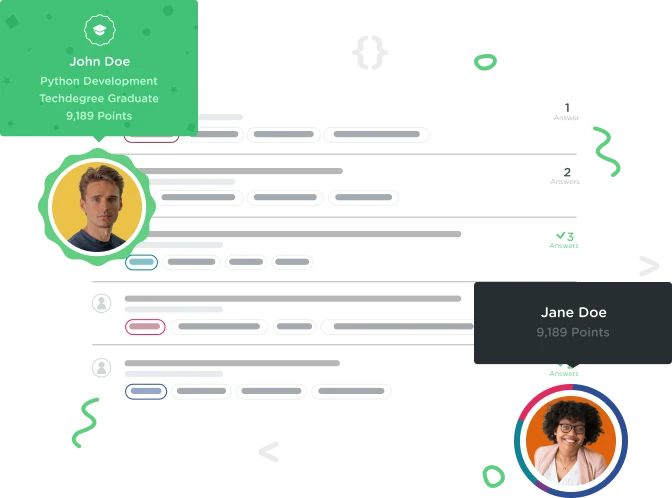

Y B
14,136 PointsDjango formset challenge - not passing
I can't seem to get past this challenge. I fixed a few errors from an earlier attempt but still no luck unfortunately.
from django.core.urlresolvers import reverse
from django.http import HttpResponseRedirect
from django.shortcuts import render
from . import forms
def product_form(request):
form = forms.DigitalProductForm()
if request.method == 'POST':
form = forms.DigitalProductForm(request.POST)
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:create'))
return render(request, 'products/product_form.html', {'form': form})
def bulk_create_products(request):
formset = forms.DigitalFormset(queryset=Digital.objects.all())
if request.method == 'POST':
formset = forms.DigitalFormset(request.POST, queryset=Digital.objects.all())
if formset.is_valid():
products = formset.save(commit=False)
for product in products:
product.save()
return HttpResponseRedirect(reverse('products:bulk_create')
return render(request, 'products/bulk_create.html', {'formset': formset})
2 Answers

Tatiana Vasilevskaya
Python Web Development Techdegree Graduate 28,600 PointsLet's go from top again. You keep writing this:
queryset=Digital.objects.all()
but what is Digital
? You have never imported Digital
, how you can access it then? This is just a question to think about, you don't need Digital
for this code challenge.
By default when you create a formset from a model, the formset will use a queryset that includes all objects in the model. You don't need to pass anything to queryset in our case as the default behaviour is already what we need.
If you change the code as described above, you will pass the chalenge. However, there is one thing I want to show you. This code
products = formset.save(commit=False)
for product in products:
product.save()
is equal to this
formset.save()
And now you can compare two views you have in your views.py
file, product_form
and bulk_create_products
. Notice how similarly you deal with forms and formsets.

Tatiana Vasilevskaya
Python Web Development Techdegree Graduate 28,600 PointsIf the method is GET, you would expect an empty formset; if the method is POST, you want to populate the formset with the POST data. Your code does this for both GET and POST, this should be corrected:
formset = forms.DigitalFormset(request.POST, queryset=Digital.objects.all())
Here you forgot the colon:
if request.method == 'POST'
Where does that come from? There is nothing of that kind in the task. Even if it was necessary, what is Digital()
?
for product in products:
product = Digital()
product.save()

Y B
14,136 PointsThanks fixed the first 2 issues. However I'm very unclear what we are meant to be doing with the saved data and how this ties back to the models?
Y B
14,136 PointsY B
14,136 PointsThanks for the tips.