Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial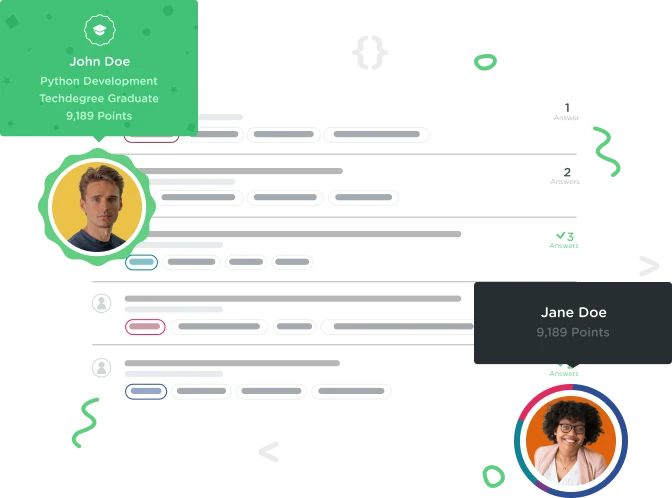
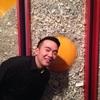
Christopher Gunawan
10,754 PointsDjango Formwizard setting initial data for forms
def get_form_initial(self, step):
if 'pk' in self.kwargs:
return {}
return self.initial_dict.get(step, {})
def get_form_instance(self, step):
if 'pk' in self.kwargs:
pk = self.kwargs['pk']
resume = Resume.objects.get(id=pk)
if step == 'resumes':
return resume
if step == 'work_experience':
work_experience = resume.workexperience_set.all()
return work_experience
if step == 'certifications':
certification = resume.certification_set.all()
return certification
if step == 'education':
education = resume.education_set.all()
return education
if step == 'skills':
skill = resume.skill_set.all()
return skill
if step == 'languages':
language = resume.language_set.all()
return language
return None
Hi! I'm currently working on my first Django project and I've run into a bit of an issue with Django Formwizard. I want to insert initial data into the forms when "editing" them. I have a url that goes like /app/edit/resume/25.
It's my understanding that the 25 or PK is pass into self.kwargs when get_form_instance is run. But for some reason, even when I don't pass in 25 or any variable through the URL (lets say when I create a new form), it still runs my code under "if 'pk' in self.kwargs'.
Can anyone shed some light on why?
I also have an issue when I am saving my forms when completed. I end up creating a new instance instead of saving the current one.
Any help would be much appreciated. Thank you so much!