Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial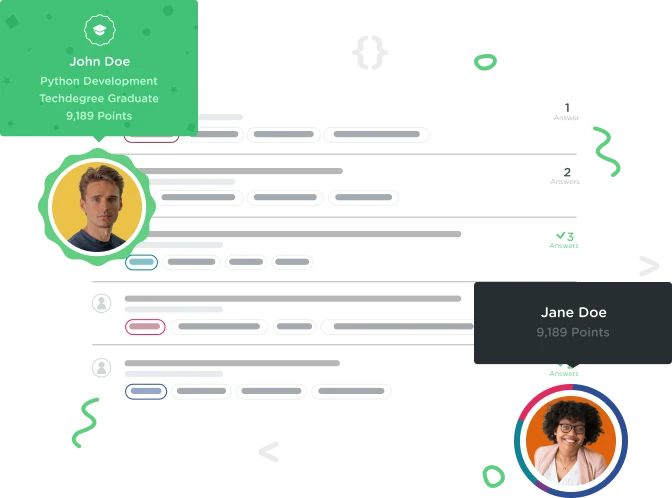
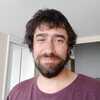
Rodrigo Muñoz
Courses Plus Student 20,171 PointsDjango: How can I retrieve a value from the middleware
I'm trying to retrieve loading times per view and displaying it in the page. To do so I'm using a middleware and a context_processor setup but I can't find the way to retrieve this values to the context of the view.
Here is the example:
I've added the middleware and context_processor to the settings.
middlewares.py:
from __future__ import unicode_literals, absolute_import
from django.db import connection
from time import time
from operator import add
import re
from functools import reduce
class StatsMiddleware(object):
def process_view(self, request, view_func, view_args, view_kwargs):
# get number of db queries before we do anything
n = len(connection.queries)
# time the view
start = time()
response = view_func(request, *view_args, **view_kwargs)
total_time = time() - start
# compute the db time for the queries just run
db_queries = len(connection.queries) - n
if db_queries:
db_time = reduce(add, [float(q['time']) for q in connection.queries[n:]])
else:
db_time = 0.0
# and backout python time
python_time = total_time - db_time
stats = {
'total_time': total_time,
'python_time': python_time,
'db_time': db_time,
'db_queries': db_queries,
}
# this works fine
print(stats)
# replace the comment if found
if response:
try:
# detects TemplateResponse which are not yet rendered
if response.is_rendered:
rendered_content = response.content
else:
rendered_content = response.rendered_content
except AttributeError: # django < 1.5
rendered_content = response.content
if rendered_content:
s = rendered_content
# The following code is commented
# because it can't compile bytes and
# as long as I understand the values comes
# from the rendered template which are the ones I'm interested in getting
# regexp = re.compile(
# r'(?P<cmt><!--\s*STATS:(?P<fmt>.*?)ENDSTATS\s*-->)'
# )
# match = regexp.search(s)
# if match:
# s = (s[:match.start('cmt')] +
# match.group('fmt') % stats +
# s[match.end('cmt'):])
# response.content = s
return response
context_processors.py
#from django.template import RequestContext
def foo(request):
context_data = dict()
context_data["stats"] = "stats"
return context_data
views.py:
from django.shortcuts import render
from accounts.models import User
def myFunc(request):
users = User.objects.all()
return render(request, 'index.html, {'users_list': users})
index.html:
{{stats}}
<br>
{% for user in users_list %}
{{user}} <br>
{% endfor %}
What is the proper way to retrieve these values?