Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial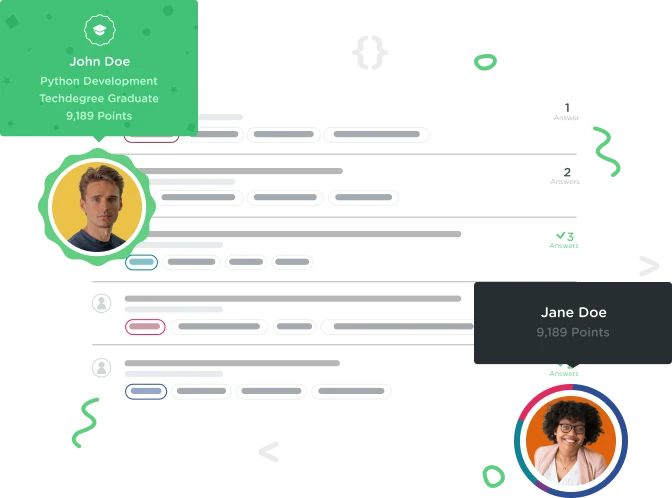

Idai Yaffe
20,429 PointsDjango SignUp view challange
I think my problem is that I can't get the user, I tried getting "username" and "password" from the post data and that didn't work.
from django.contrib.auth import authenticate, login
from django.core.urlresolvers import reverse_lazy
from django.views import generic
from . import forms
class SignUp(generic.CreateView):
form_class = forms.UserCreateForm
template_name = 'accounts/signup.html'
success_url = reverse_lazy('products:list')
def form_valid(self, user):
username = self.request.POST['username']
password = self.request.POST['password']
user = authenticate(username=username, password=password)
if user is not None:
if user.is_active:
login(self.request, user)
return super().form_valid(form)
1 Answer
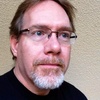
Chris Freeman
Treehouse Moderator 68,457 PointsYou are very close. I had to research the answer. See Lewis Cowles' answer on Oct 6, 2016 (from this thread]
In brief, add a line at the top of the method:
res = super().form_valid(form)
Then change the return
statement to:
return res
Idai Yaffe
20,429 PointsIdai Yaffe
20,429 PointsI have changed my code, I think this might be closer to the solution but the code doesn't work still.