Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial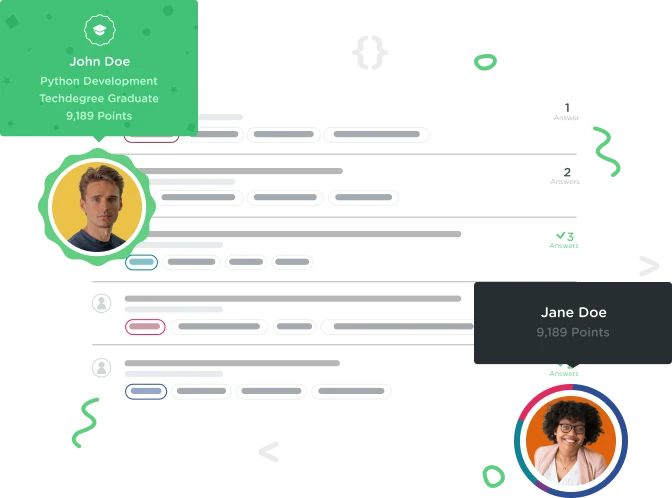

Rachel Vasquez
Courses Plus Student 887 PointsDo both task functions need to work together and from the same list? Help?
I think I'm confused - for task 1 and 2, it's not clear to me whether both functions are using the same list.
The first task is to create a function with one parameter that adds to a list and we need the sum of that list.
The second task for another function seems like it's asking to do the same thing as the first task, except adding a string to the printed or returned total? So was task 1 for the sake of a building block and then task 2 is an expanded version of task 1? Meaning these functions have nothing to do with one another?
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
my_list = []
def add_list(item):
my_list.append(item)
for item in my_list:
return sum(item)
def summarize(item):
my_list.append(item)
for item in my_list:
total = sum(item)
return "The sum of {} is {}".format(my_list, total)
6 Answers

Alex Carlson
600 PointsI believe there's an issue in your first function that's holding you up. From what I can see, it looks like you're adding the items to your list, then iterating through the list and summing each individual item in the list. So, for example, if your list had 1, 2, and 3, as written, your for loop would go to index 0, sum the number 1, and return the number 1. Then move on to 2, then 3 and so on.
Instead, you could use Python's sum function to sum the entire list at once and return it. Using this method, your add_list function would look like this:
def add_list (listToAdd):
'''Use the sum function to find the sum of the list.
Type "help(sum)" into the Python console for more info on usage'''
summedList = sum(listToAdd)
return summedList
# End of first function
For your second function, you could then call your first function as Bart suggested. This would look like:
def summarize (listToSummarize):
# To get the total, or the X in the challenge, use your answer to question 1 to find the sum of the list
total = add_list(listToSummarize)
# Then, create a string variable to return
stringToReturn = "The sum of {} is {}".format(listToSummarize, total)
# Finally, return that string at the end of the function
return stringToReturn
# End of second function
This should run in both the workspace and the quiz. Hope this helps!
-Alex
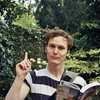
Bart Bruneel
27,212 PointsHello Rachel,
You could write the two functions independently and then write the addition-part two times (as you have done) or you can call the add_list function in the summarize function to first make the addition and then place the total in the string. However, the sum function is used on the whole list as in sum(my_list). If you cannot use the sum function you can write a for loop that loops over each item in the list and then append it to a total that you have set to zero before the for-loop. Like this
total= 0 for item in my_list: total+=item

Rachel Vasquez
Courses Plus Student 887 PointsHmm, the counter makes sense, but it looks like the quiz gives me an error. It says "Unsupported operand type +=: 'int' and 'list'. I left the first function as is, and edited the second like this:
def summarize(item):
my_list.append(item)
total= 0
for item in my_list:
total+=item
#total = sum(item)
return "The sum of {} is {}".format(my_list, total)
Man, I think this is the first time I've gotten stuck on one of these quizzes so far haha. :-) I'm not sure what I'm doing wrong and having trouble running it on Workspaces. I wish there was a var_dump-ish kind of function for Python because sometimes the errors are vague... is there?
I think one the the reasons I'm confused is normally a "for" loop like in PHP, a for each, goes through each individual item and does something with each item. I don't really get why I'd need a "for" loop to add these items - or maybe that's my mistake? I don't need one for the summarize function?
-x-x-x-x-x- UPDATE BELOW -x-x-x-x-x-
Okay so the for loop didn't make sense to me in summarize so I did this instead:
def summarize(item):
all_items = my_list.append(item)
total = sum(all_items)
return "The sum of {} is {}".format(my_list, total)
To me, this is append items to a list and save into all_items variable. Then take all_items and get the sum, save that to a total variable. Then return the string that calls both my_list and the total variable. Now the error says, "NoneType object is not iterable". No idea what that means.
Think I'll come back to this later...

Alex Carlson
600 PointsI had trouble creating the string in the return statement. I'm sure there's a smarter way to do this, but I just created a variable above the return statement that held the string with the formatting:
stringToPrint = "The sum of {} is {}.".format(theString, total)
Then, I made the last line of the function:
return stringToPrint
That fixed it for me.

Rachel Vasquez
Courses Plus Student 887 PointsDid you use the original code I posted in the question? Want to test it out in the workspaces before I run it on the quiz.
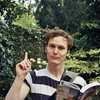
Bart Bruneel
27,212 PointsHey Rachel,
my_list is the argument of the function. So if you want to use the function add_list inside summarize_list you'll also need to pass the argument my_list to add_list. Imagine for example, if you would have multiple nested functions that take different number of arguments inside one function. If you would pass no arguments to the nested functions the functions wouldn't know what to work on.
The same holds true if you don't pass any arguments to summarize_list. When you call the function yourself (by putting summarize_list(my_list)) after the function definitions, the program first looks for the function summarize_list and passes to this function the argument my_list, which then get's passed on to the function add_list.

Rachel Vasquez
Courses Plus Student 887 PointsHi Bart,
Ah okay so in this case, it is the two functions just passing the list to each other so the second function could make use of it in add_list. Cool - thanks for explaining that. :-)
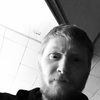
Kody Dibble
2,554 PointsSo it gave me a error from the second function 'the sum of [[1,2,3],[1,2,3]] is 6.
Not sure what's up I used the function you called? Where is getting two of the 1 2 3 statement?

Alex Carlson
600 PointsHi Kody,
Could you please repost your code so I can take a look?
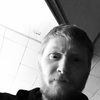
Kody Dibble
2,554 PointsI lost the code lol but it was basically hers and yours together.
Rachel Vasquez
Courses Plus Student 887 PointsRachel Vasquez
Courses Plus Student 887 PointsThis is exactly what I needed, a walk through. So a "for" loop wasn't even necessary - which is misleading because the instructions on the quiz suggest that you may need one. I think that's the part that kept confusing me the most. I wish they'd just given the task without suggestions in case the person doing the quiz finds another way to do it and I didn't have to append items either.
So you can literally place the variable of an empty list into a function as an argument? This is what I wrote that finally completed the quiz.
The only part I don't really understand is why we have to repeat my_list in both functions? Is it just for the sake of being able to re-use it in the second function without any weird scope problems? Like why not just write def_summarize() with nothing in parenthesis and then the rest of the function normally?
Thanks so much for the help, I appreciate it. :-)