Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial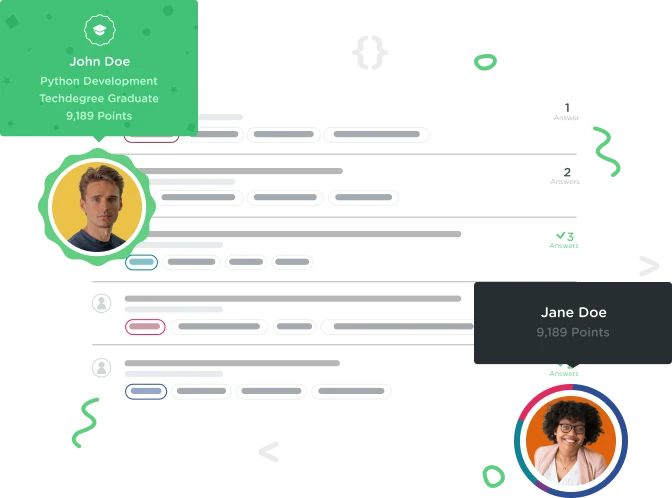

mattlebl
10,849 PointsDo functions have to be instantiated and then declared?
On Treehouse I'm taught to declare functions like this:
void someFunction();
int main() {
return 0;
}
someFunction() {
}
Do I have to declare the function up top first, or can I just instantiate and white the function at the same time?
Edit: Whoops, declare then implement, my bad. I got the stupid jargon mixed up. :D
2 Answers

Stone Preston
42,016 PointsIn C, functions must be implemented before they can be called unless you provide a function prototype first (also known as declaring the function).
Below is an example of implementing a function before its called:
//provide the implementation before calling the function in main
int someFunction(int a, int b) {
return a + b;
}
int main() {
int a = someFunction(1,2); //call the function implemented above. this works since the compiler already knows about the function since its fully implemented before this call is made in the file
}
below is an example of providing a function prototype, calling the function, and implementing it below the main method after its called:
//this is the prototype or function declaration
int someFunction(int a, int b);
int main() {
int a = someFunction(1,2); //call the function declared above. this works because the compiler saw the prototype you declared up above before calling the function
}
int someFunction(int a, int b) {
return a + b;
}
here is an example of something that would not work. calling a function that does not have a prototype declared with its implementation down below the function call
int main() {
int a = someFunction(1,2); //call the function. there is no prototype and the function is implemented down below after the funciton call. this will give you undefined behavior/errors
}
int someFunction(int a, int b) {
return a + b;
}
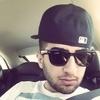
Vrezh Gulyan
11,161 PointsYou can do what is called prototyping which is when you let the program know that you will be declaring a function later after main just so you can use it inside of the main function. You can do what the previous person said which is declaring the function before main so you don't have to prototype but it is more common practice to prototype as main is expected to be the first function of a program.
mattlebl
10,849 Pointsmattlebl
10,849 PointsGreat. Thank you.
Jose Ramos
1,908 PointsJose Ramos
1,908 PointsFantastic example there, thank you. Good to know that you can do the first one.