Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial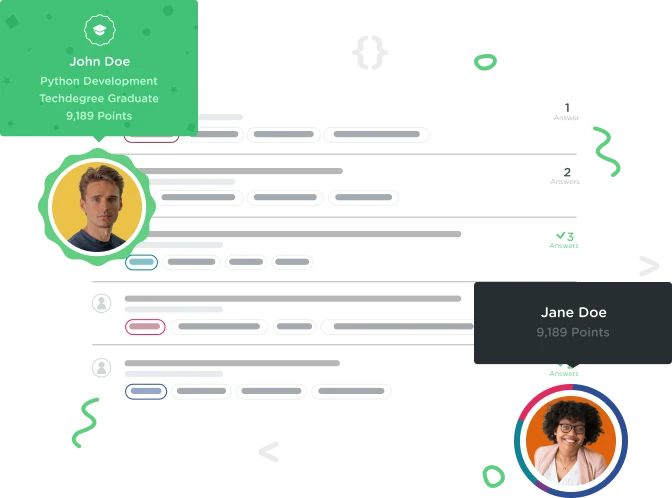
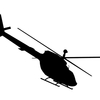
Timothy Riordan
506 PointsDo I have loops where I don't need them?
I guess I am just trying to clean up my coding skill a little. Now compared to Kenneth Love code for this solution mine is a little simplistic, but similar I guess. The question I have is, do I have while loop where I don't need one? Namely the second one in my code (see below)? Should I have used else rather than elif etc...
import random
random_num = random.randint(1, 10)
number_of_guesses = list()
allowed_guesses = 5
while len(number_of_guesses) < allowed_guesses:
print("I am thinking of a number 1 - 10, can you guess it?")
user_guess = input("> ")
number_of_guesses.append(user_guess)
if int(user_guess) == random_num:
print("CONGRATS! You guessed it")
break
elif int(user_guess) != random_num:
print("No thats not it. You have used {} guesses out of 5.".format(len(number_of_guesses)))
continue
while len(number_of_guesses) == allowed_guesses:
print("You have ran out of guesses")
print("My number was {}.".format(random_num))
break
Also just wanted to say thank you to Kenneth Love and everyone at Treehouse and the forums for creating the Python3 course.
Tim
4 Answers
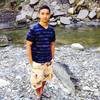
Nazmus Sarwar
5,625 PointsThe while loop at the end was unnecessary.
An if loop inside of your main while loop would have been sufficient enough to end the loop.
import random
random_num = random.randint(1, 10)
number_of_guesses = list()
allowed_guesses = 5
while len(number_of_guesses) < allowed_guesses:
print("I am thinking of a number 1 - 10, can you guess it?")
user_guess = input("> ")
number_of_guesses.append(user_guess)
if number_of_guesses == allowed_guesses:
print "You have ran out......."
print "my number was....".format(...)
break
elif int(user_guess) == random_num:
print("CONGRATS! You guessed it")
break
elif int(user_guess) != random_num:
print("No thats not it. You have used {} guesses out of 5.".format(len(number_of_guesses)))
continue
It is also better to have a counter than to make an array. So:
i = 0
and every time you loop through the while loop (not the for loop), you add 1 to i. (i +=1)
so it would be
if i == 5:
print "You have ran out......."
print "my number was....".format(...)
break
much easier to read and less jargon.
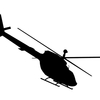
Timothy Riordan
506 PointsNazmus,
Thank you for the help. I guess I over thought the second while loop, it looks much cleaner the way you have presented it. I am still a little confused on how to make the counter work, something I will have to look up and try.
Again, thanks for help.
Tim
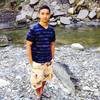
Nazmus Sarwar
5,625 PointsThe counter is pretty simple! Outside of the while loop, you set i = 0 (this can actually be any variable, but loop variables are "i" by convention. Inside the while loop, the "counter" part is when when you add i = i + 1 or i+=1 at the end of the loop so you your i = 0 that you set increases by one every time you go through the loop (ie. each time the user guesses). Also, by logical flow, you want to have this as the first if statement so you can properly break the while loop (since if another condition was true, you would never get to the if loop that breaks out.
i = 0
while True:
if i >= 5:
break
#code such as other logical operators and if statements and elifs
i = i + 1 #or i +=1
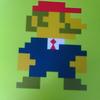
KAZUYA NAKAJIMA
8,851 PointsNazmus, In video, teacher Kenneth uses list to count times of guess. I thought count by enumerate like 'i' value is simpler. is there any specific reason for using list to count guessing times? I'm just curious.