Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial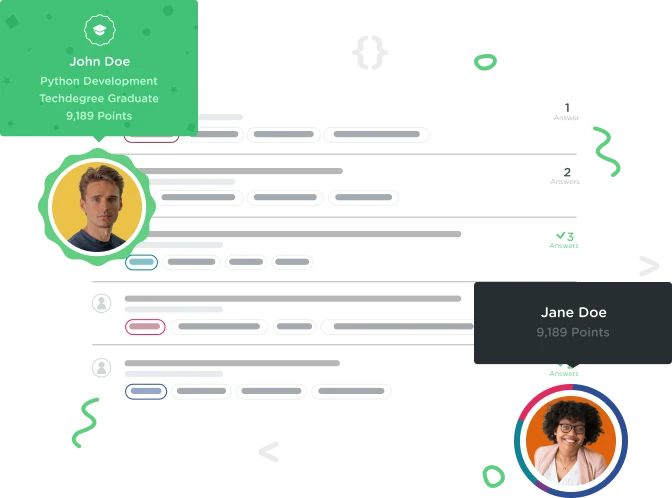
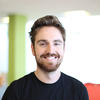
Kristian Woods
23,414 PointsDo I understand the concept behind classes, getters and setters in Javascript?
ok, so I just want to go over the concept of javascript classes, setters, getters, static and shared methods
class Person {
constructor(name) { // create a name propeerty and store the value in newly created obj/instance (bob)
this.name = name;
}
set age(ageValue) { // create a setter - age is now a property.
let myAge = ageValue;
return myAge;
}
static addYear(year) { // static method only accessed by Person.
this.age += year;
}
get getName() {
return this.name; // your getter name (getName) CAN'T be named the same as the property your're trying to return (this.name) // returns the value given to the newly created instance of the class.
}
}
Person.age = 20; // setting age
const bob = new Person('bob'); // create new instanace of Person
Person.addYear(68); // adding to age with static method
console.log(Person.age); // print newly set age to console
console.log(bob.getName); // print name property to console
The setter creates a property named 'age'
The static method adds a year on to the 'age' property. This static method is only available to the Person class, and not any instances of the class.
The getter returns the name value given to the instance of the class.
So, essentially, getters and setters are just properties?
Why would a static method be useful?
Thanks
1 Answer

Alexander La Bianca
15,959 PointsThe getters and setters in javascript are here in an attempt to mask javascript as an object oriented programming language like java or c#. In those languages it is common to keep your properties private and then create public setters and getters to access these properties. Now javascript does not have private properties like other languages do, but we can still follow the convention. Let me explain by going over your Person class
//It is a common convention to name 'private' properties with an underscore like the below _name
class Person {
constructor(name) { // create a name propeerty and store the value in newly created obj/instance (bob)
this._name = name;
this._age = 0; //also give it an age properties as you have your setters and getters for it (give it a default of 0)
}
set age(ageValue) { // create a setter - age is now a property.
this._age = ageValue; //set the private property _age
}
static addYear(year) { // static method only accessed by Person.
this.age += year;
}
get name() {
return this._name; //now you can get the private _name. Now you wonder why a getter and setter are needed?
//Imageine instead of just returning the _name as it is you do
return this._name.toUpperCase(); //now you will always return the upper case value.
}
set name(nameValue) {
this._name = name;
}
}
const bob = new Person('bob'); // create new instanace of Person
Person.addYear(68); // adding to age with static method
console.log(bob.name); // print BOB to the console.
bob.name = "Alex"; // calls the set name(value)
bot.age = 25; //calls the set age(value)
To answer your question about static methods. Static methods are methods you can call on your class without having to instantiate an instance first. Math.min() and Math.max() are such examples.
In your case addYear(year ) is the static method so you can call Person.addYear() without having to do:
const bob = new Person("bob")
bob.addYear(20);
However, be careful when you use static methods and modify instance properties like you do in addYear