Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial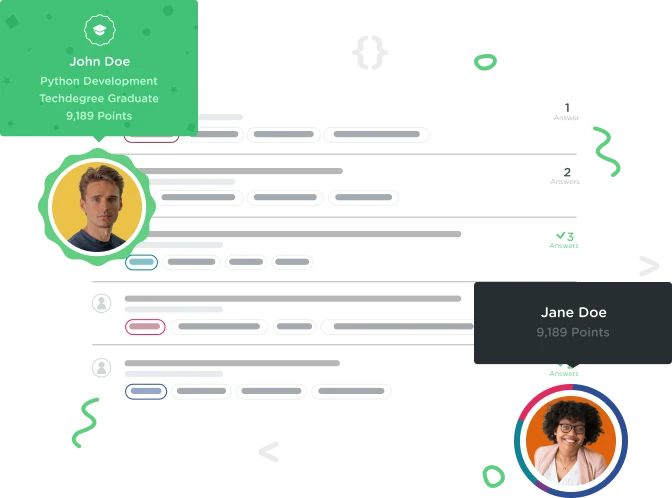

matt anderson
Full Stack JavaScript Techdegree Student 10,777 PointsDo Nested loops need an array in the top level loop?
In the top for loop we create an array called columns that is empty. Then in the nested loop we create a variable which we push in to the columns array. Then we push the columns array into the space array. Why do we need the columns array? Is the columns array somehow a helper to get information out of the loops?
3 Answers

Mathew Gries
7,072 PointsFor anyone having trouble understanding what is going on here, what you are building is a 6 x 7 grid. The first array declared in the method is the complete grid: const spaces = [] When spaces is finished being built, it will be an array of arrays, meaning each index in spaces will be an array itself. Each one of these arrays hold a collection of Space objects, each Space object representing a column in that row.
spaces = [
[Space(0,0), Space(0,1), Space(0,2), Space,(0,3), Space(0,4), Space(0,5), Space(0,6)], // First row
[Space(1,0), Space(1,1), Space(1,2), Space,(1,3), Space(1,4), Space(1,5), Space(1,6) ], // Second row
[Space(2,0), Space(2,1), Space(2,2), Space,(2,3), Space(2,4), Space(2,5), Space(2,6) ], // Third row
{/*...Continue patter for three more rows*/}
]
I do believe she may have had the loop conditions backward. The outer loop should be
// Each outer loop should represent a row
fo(let x = 0; x < this.rows; x++)
And the inner loop should be:
//Each inner loop should represent the columns in that row
for(let y = 0; y < this.columns; y++)
So, when we enter the first loop, we will be working on each row. Then when we enter the second loop, we will be creating each column in the current row.
The flow should be this:
for(let x = 0; x < this.rows; x++){
// We enter the first loop
// This will represent a row on our board
// We create an array to hold the columns for this row
// Every iteration of this first loop will create a new instance of this variable
// The previous instance will be overwritten each time
const columns = [];
for(let y = 0; y < this.columns; y++){
// We enter the second loop. This will iterate seven times before exiting
// Each iteration will create a column, or Space, for the current row of our first loop
const space = new Space(x,y);
// Push the column, or Space, to our columns array
columns.push(space);
}
// We have exited the second loop after seven iterations, and are now back in the first loop
// We are still in the same iteration of the first loop from when we first entered.
// Push the columns array from our second loop into the spaces[] array
// This creates one row in our 2D array.
// See the example above, the first iteration creates the Spaces who's indexes start with 0, Spaces(0,1),
// Spaces(0,2). Etc
spaces.push(columns);
// We have reached the end of our first loop. If we have not met the condition to exit the loop, we will
// Go back to the top and start over, creating our next row in the spaces array
}
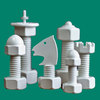
Steven Parker
231,269 PointsThis structure demonstrates a technique for creating 2-dimensional arrays. Each item of the "spaces" array is a "column" array which contains all the space objects in a single vertical column. All of them together ("spaces") represent the entire board.
The nested loops provide a handy way to build up the board in both dimensions.
As the project continues, you will see how two indexes (one for each dimension) are used to access a particular space.
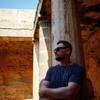
mersadajan
21,306 PointsAt first, I had two for loops to create a one dimensional array of spaces, but this doesn't make much sense if you want to easily access each space later. You need arrays in an array to create a kind of coordinate system. I guess, later we will be able to access each space by just using something like spaces[x][y] where is x would be the column and x the row we choose.
Ricardo Leitão Pedro
6,219 PointsRicardo Leitão Pedro
6,219 PointsI don't think so, and I actually tested inverting the logic as you proposed which confirmed my impression. It makes sense to build the grid or board in the way she put it, if you think about it as you would on a standard algebra graph: as you move horizontally (through the x axis) you populate the columns, whereas moving vertically (through the y axis) you populate the spaces in each column by going through the rows.