Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial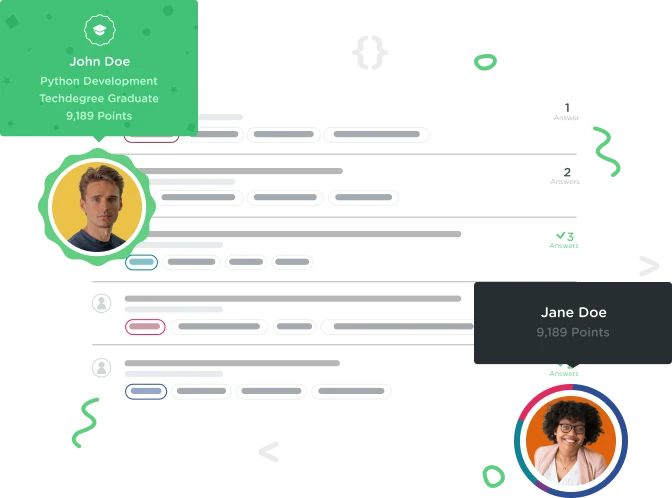
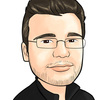
Dani Ivanov
10,732 PointsDo something only the first time a for loop executes
So I want to create a hashtags (#) triangle using a for loop and the result should be something like this:
#
##
###
####
#####
######
#######
########
#########
##########
I have this simple loop and it works just fine.
var holder = "";
for (var q = 0; q < 10; q++) {
holder += "##";
console.log(holder);
}
I added two hashtags on each iteration so that the triangle appears more flat, but I want the very first time the loop is executed to log only a single hashtag and then two from there on.
So I added an if statement like this.
var holder = "";
for (var q = 0; q < 10; q++) {
if(q === 0) {
holder += "#";
continue;
}
holder += "##";
console.log(holder);
}
But the if statement seems to evaluate to true every time the for statement is executed.
What am I doing wrong?
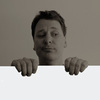
Sean T. Unwin
28,690 PointsGood tip, Robert Richey.
I just wanted to clarify, as well, that when Dani Ivanov states:
But the if statement seems to evaluate to true every time the for statement is executed.
What am I doing wrong?
it is because an else
clause is needed for further checking and also that continue
needs to be removed as it is essentially cancelling out the if
statement in this case.
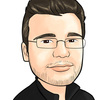
Dani Ivanov
10,732 PointsThanks for your responses. I fixed it by adding the console.log line before the continue statement. Actually that is what I was trying to do. Sometimes I make the most stupid mistakes :)
You mentioned that you are reading other developer's code to help you solve problems you are having at the moment. Do you read the whole thing or just the snippet that tackles the problem you are facing? If just the snippet, then how do you find that snippet amongst a huge file of JavaScript code?
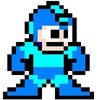
Robert Richey
Courses Plus Student 16,352 PointsDo you read the whole thing or just the snippet that tackles the problem you are facing? If just the snippet, then how do you find that snippet amongst a huge file of JavaScript code?
It's normally a fundamental problem that's easy to Google and read about on Stackoverflow - like looking up an error code or which for loop to use in JavaScript - for, for..each, for..in, etc. But the way some developers solve these errors or use loops are a little or a lot different than what I'm used to seeing, like whether to use a pre-increment or post-increment on your index variable when setting up a for loop; stuff like that.
After a while, it's hard to remember some differences between languages, so I stopped trying and just use Google when I need it.
1 Answer

fitri
Front End Web Development Techdegree Student 10,450 PointsYou're going to start with "###" on the first line. Because: Your condition is true so the first iteration will concatenate "#" and then continue to concatenate "##". To fix this you need to add an else statement so your loop will either concatenate "#" or "##" instead of performing both. You also don't need continue and the statement is actually false on the other iterations.
var holder = "";
for (var q = 0; q < 10; q++) {
if(q === 0) {
holder += "#";
} else {
holder += "##";
}
console.log(holder);
}
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Dani,
There's also a way to fix your code by adding another console.log before the continue statement.
Here's another way to consider writing this - the if conditional will be true for every step but the first, reducing the lines of code being evaluated. I present this just as another way to go about solving this problem - I learn a lot by reading how other developers write their code to problems I'm working on.