Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial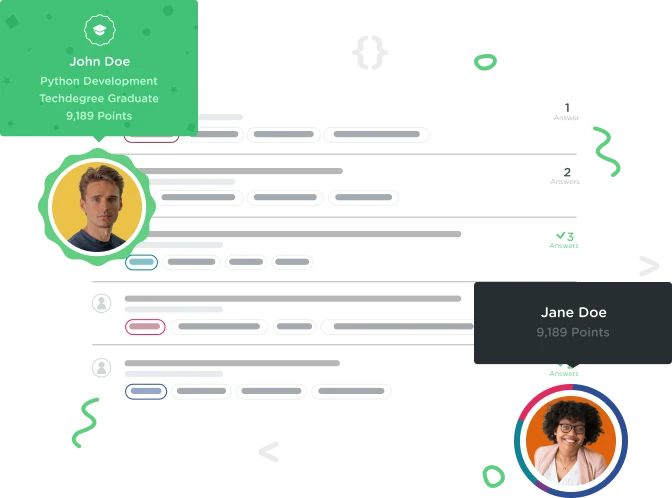
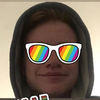
Nils Garland
18,416 PointsDo we even need the getter here? And why is it there?
Hi, just speeding through the new Java objects course, and I found something.
If you have never taken the course, or you've forgotten what it was. We are making a fictional pez dispenser. So, when we instantiate our class we have a controller that takes a string, the string is called characterName and is used to set the character head on the dispenser.
Above the controller however we have a variable. And it's called characterName, the variable is public and final, so it can't be accessed or changed unless when we instantiate it of course.
But then, why do we need a getter method? If the variable was private I would understand, but it's public. So why not just set the variable to final and get the variable instead of using the getter method?
Strange, not an issue but I'm just curious.
Class file:
// Class
class PezDispenser
{
// Variables
public static final int MAX_PEZ = 12;
public final String characterName;
// Constructor
public PezDispenser(String characterName)
{
this.characterName = characterName;
}
// Methods
public String getCharacterName()
{
return characterName;
}
}
Execution file:
public class App
{
public static void main(String[] args)
{
System.out.println("Welcome!");
PezDispenser dispenser = new PezDispenser("Yoda");
dispenser.characterName = "Darth Vader";
System.out.printf("The character is %s! %n", dispenser.getCharacterName());
}
}