Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial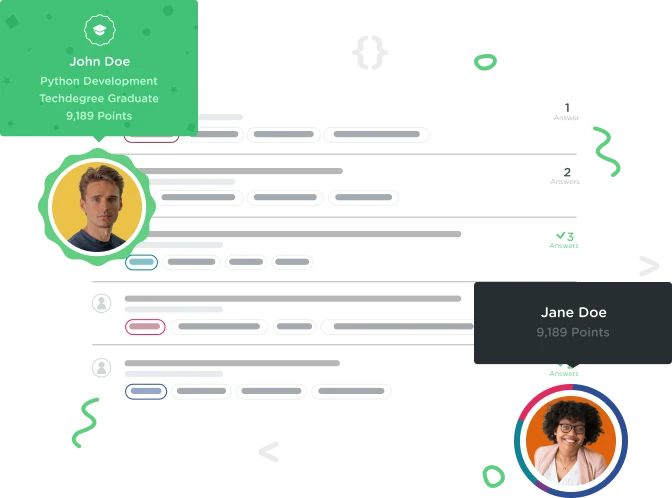
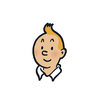
Yeeka Yau
7,410 PointsDo we need to create a new DialogFragment class for each Alert?
Hi, this might be a dumb question, but in this app, we have created a new class: AlertDialog Fragment which extends the DialogFragment class. It seems that we override the onCreateDialog method in order to display some different text for an alert dialog.
So when the network is unavailable, instead of a toast, I have created another Dialog, by creating a new class as done previously. Do we need to do this for each new Dialog? It seems like it a bit of overkill - for what seems to be just a fairly simple task of displaying a message..Is there a simpler way to just change the text of the dialog?
Thanks in advance!
4 Answers

Dan Johnson
40,532 PointsIf you're just changing something like text content without adding additional behavior, it is usually better to keep it as one class as you were finding out. Here's one way to add variable messages and titles to the dialog used in the weather app:
public class AlertDialogFragment extends DialogFragment {
//Supply keys for the Bundle
public static final String TITLE_ID = "title";
public static final String MESSAGE_ID = "message";
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
//Get supplied title and message body.
Bundle messages = getArguments();
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setPositiveButton(context.getString(R.string.error_ok_button_text), null);
if(messages != null) {
//Add the arguments. Supply a default in case the wrong key was used, or only one was set.
builder.setTitle(messages.getString(TITLE_ID, "Sorry"));
builder.setMessage(messages.getString(MESSAGE_ID, "There was an error."));
}
else {
//Supply default text if no arguments were set.
builder.setTitle("Sorry");
builder.setMessage("There was an error.");
}
AlertDialog dialog = builder.create();
return dialog;
}
}
Then you could set them on creation:
private void alertUserAboutError() {
Bundle messageArgs = new Bundle();
messageArgs.putString(AlertDialogFragment.TITLE_ID, "New Title");
messageArgs.putString(AlertDialogFragment.MESSAGE_ID, "New Message Body.");
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.setArguments(messageArgs);
dialog.show(getFragmentManager(), "error_dialog");
}
If this was a class that didn't have to deal with removal/restoration of views you could just supply a new constructor to do this.
And of course you'd want to add arguments to alertUserAboutError if you wanted to make it dynamic.

Benyam Ephrem
3,185 PointsI did a good amount of research on this because I had the same question as you... as far as I know it is very likely that you must create separate classes for different dialog boxes, after all, a class is a blueprint so if you want different dialog boxes you likely need a different blueprint to build different boxes......which makes sense. An app most likely won't have THAT many alert dialog boxes so I don't really see making a new class for each fragment as problem as long as you name the classes in a way that makes it easy to know which alert is which.
If you do find a way to do it please tell me because I'd like to know :)
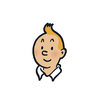
Yeeka Yau
7,410 PointsThanks Ben, seems like we got our answer below.

Javier Delgado
Courses Plus Student 509 PointsHi, the answer to this question has helped me a lot, but I'm trying to figure out how I can pass the correct arguments to alertUserAboutError()... I'm a complety newbie on Java. Any help is greatly welcome! Thanks!

Dan Johnson
40,532 PointsIf you wanted alertUserAboutError to have arguments you could change it to look like this:
private void alertUserAboutError(String alertTitle, String alertBody) {
Bundle messageArgs = new Bundle();
// Use the arguments to set up the bundle
messageArgs.putString(AlertDialogFragment.TITLE_ID, alertTitle);
messageArgs.putString(AlertDialogFragment.MESSAGE_ID, alertBody);
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.setArguments(messageArgs);
dialog.show(getFragmentManager(), "error_dialog");
}
And then call it somewhere within your class like this:
alertUserAboutError("My Title", "My Message");

Javier Delgado
Courses Plus Student 509 PointsThank you so much Dan! I've missed to say thank you, sorry!
Yeeka Yau
7,410 PointsYeeka Yau
7,410 PointsThat's awesome Dan, learnt something new. Thanks!