Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial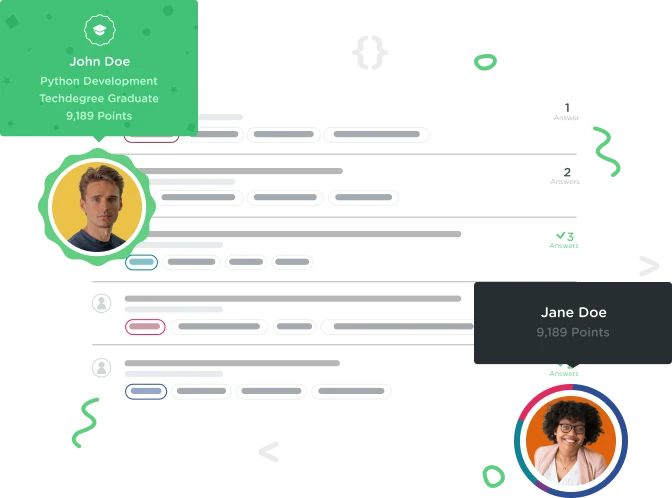
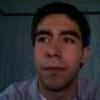
David Trejo
19,914 PointsDo... While Loop Challange
I am getting the following error in the challenge. "There was an error with your code: SyntaxError: Parse error". I tried using jshint.com and jsbin.com to see where the syntax error is but I am unable to find it. I was wondering if anyone can help me out of this one. What could be the Syntax problem in this script?
Code:
var secret; var guessCount = 0; var correctGuess = false;
do { secret = prompt('What is the secret password?'); guessCount += 1;
if(secret === 'password'){
correctGuess = true;
}
while(!correctGuess){
document.write('You know the secret password Welcome');
}
}
3 Answers
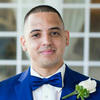
Justin O'Reilly
3,546 PointsYou say you have:
var secret; var guessCount = 0; var correctGuess = false;
do { secret = prompt('What is the secret password?'); guessCount += 1;
Have you tried:
var secret; var guessCount = 0; var correctGuess = false;
do { secret = prompt('What is the secret password?');
guessCount += 1;
Basically moving the guessCount +=1; to a new line since you have closed the statement with the semi colon JavaScript isn't looking at anything on that line after it.
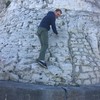
Jimmy Saul
9,851 PointsThere will definitely be more experienced people out there than I who can answer this for you (and please correct me) but I think that for the do.. while loop to keep iterating through the code the condition of 'while' needs to evaluate to true, and maybe one problem here is that var correctGuess = false, but the while condition is (!correctGuess) so, NOT correctGuess, i.e. they don't match
This is my attempt at solving it...
var guessCount = 0;
do {
var secret = prompt('What is the secret password?');
guessCount += 1;
}
while (secret !== 'password');
if (secret === 'password') {
document.write('You know the secret password Welcome');
}
document.write("<br> You took " + guessCount + " guesses");

Jacob Mishkin
23,118 PointsYou guys are all really close on this! but you have added some unnecessary conditional statements in the code. First you have to know that these code challenges are very particular. To pass the challenge you can't add anything outside of the scope of the question presented. One way to think about Do While loops is this:
do {
//do something here i.e. code here
}
while (//loop back to the code in "do" until the statement is false very similar to an if statement);
So in this challenge the first thing to see is that the var secret needs to be an empty var outside of the loop because you want the prompt function in the loop. So when your code is in the do section of the loop it will run that code first, in our case a prompt appears asking for a string. After we input the string into the prompt then the conditional statement is assigned to the string we just inputted into the var secret. It checks against the conditional statement: (secret !== "sesame") if the string is not "sesame" the loop will go back to the do section until the condition is false. In this case if the input is sesame then the condition has been met to exit the loop. where I think you are getting confused is in do while loops the condition is the "while" section of the loop. It acts just like an if statement. please see the code below. I hope this helps.
var secret;
do{
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
David Trejo
19,914 PointsDavid Trejo
19,914 PointsI am still getting the same error whether or not I move guessCount += to a new line. I don't think having two closing statements in the same line is not the problem. You can have two closing statements in a same line. For example, in a for loop you have three statements being made in the condition. This for loop will run without any error regardless if you have those three statements in three separate lines.
for(i = 0; i < 10; i++){ document.write(i); }
for(i = 0; i < 10; i++){ document.write(i); }
As for my problem, I am still kinda clueless what the solution might be. 0.o