Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial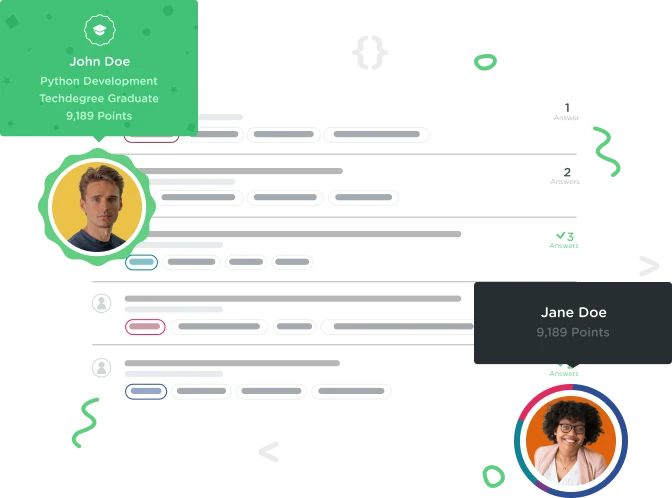

Jules Al
1,096 PointsDo While Loop conditional statement question...
Please see the conditional statement in the while loop. If correctGuess is already set to false, why would I need to place an exclamation mark next to correctGuess? I thought we want the loop to run while correctGuess is false.
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('Guess a number between 1 & 10');
guessCount += 1;
if (parseInt(guess) === randomNumber){
correctGuess = true;
}
} while ( ! correctGuess )
document.write('<h1>You guessed the number!</h1>');
document.write('It took you ' + guessCount + ' tries to guess the number' + randomNumber);
2 Answers

Galen Cook
2,463 PointsThe !correctGuess
is telling the program to keep running the code within the while loop as long as correctGuess is false. The !
just means not, so it's basically saying 'while NOT correct guess, keep running'

Jules Al
1,096 PointsOkay that's what I was misunderstanding. Thanks, everyone!
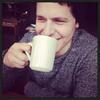
Brian Mendez
7,734 PointsYou can also do:
while ( correctGuess == false );
until you get more comfortable with '!'
Jules Al
1,096 PointsJules Al
1,096 PointsBut isn't correctGuess already set to false at the top of the code when it's declared as a variable?
So why wouldn't we just do the following...?
while (correctGuess)
I thought (!correctGuess) would evaluate to true.
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsThe while loop will only continue if the expression evaluates to true. Your code would end the loop when correctGuess is false because when you substitute the value you get
while(false)
. That is why you need to invert the condition with !correctGuess (ie. NOT(false) equals true).