Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial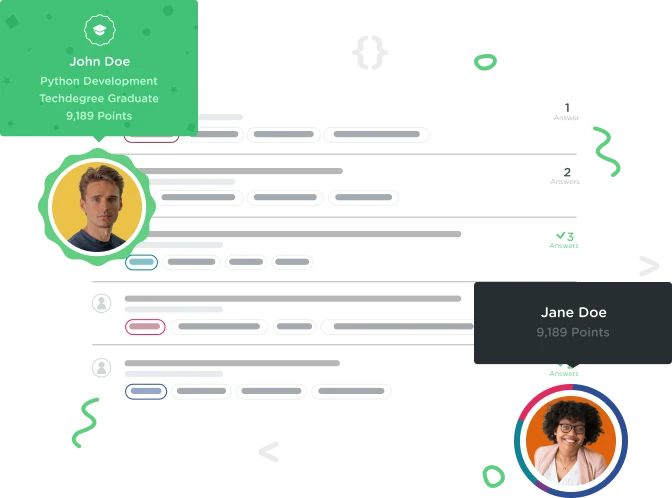

Michael Partridge
7,129 PointsDo you mean private?
This is meant to be feedback on the exercise, but I can't seem to find a way to submit feedback after having already bypassed that screen so I am doing it here. In this exercise you say to make the member variable public when we just learned that the best practice is to make them private and access them through getter and setter methods.
public class Spaceship {
public String mType;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
1 Answer
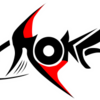
Justin Black
24,793 PointsThis is definitely an error in the videos, sadly it happens sometimes. And here is why it's best practice to declare these as private:
It breaks encapsulation - the core principle here is that the fields of your class are implementation details, by exposing it as a public field, you are telling everything outside that class that it is an actual piece of data that is stored by the class - something external classes don't need to know, they just need to be able to get or set that piece of data. As soon as you make something public, external classes should be able to depend on it, and it should not change often, by implementing as a method, you are retaining the flexibility to change the implementation at a later point without affecting users of the class.
If you wanted to change one of the fields so it's calculated, or retrieved from a service when its called, you wouldn't be able to without breaking other parts of your application. Other reasons are that it allows you to control access to the variable (i.e. make it immutable). In setters you can also add checking code, and you can add behaviors to getters and setters that do other things when a variable is get or set (though this may not always be a good thing). You can also override a method in a derived class, you can't override a field.
There are some very rare situations where it is better to have public field instead of a method - but this really only applies in very high performance applications (3D games & financial trading applications), unless you are writing one of these, avoid public fields - and if you do care about performance to that level, Java is probably not the best choice anyway.
Just a note on mutability - in general you should try to make things immutable until you have a genuine reason to make them mutable - the less your code exposes in its public API, the easier it is for long term maintenance, and in a multi-threaded situation, immutable objects are threadsafe, mutable objects need to implement locking.
Michael Partridge
7,129 PointsMichael Partridge
7,129 PointsLuckily they only got it wrong in the challenge and presented it correctly in the videos. Even so this answer was very detailed and helped me further understand the reasoning behind the practice. Thank you!