Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial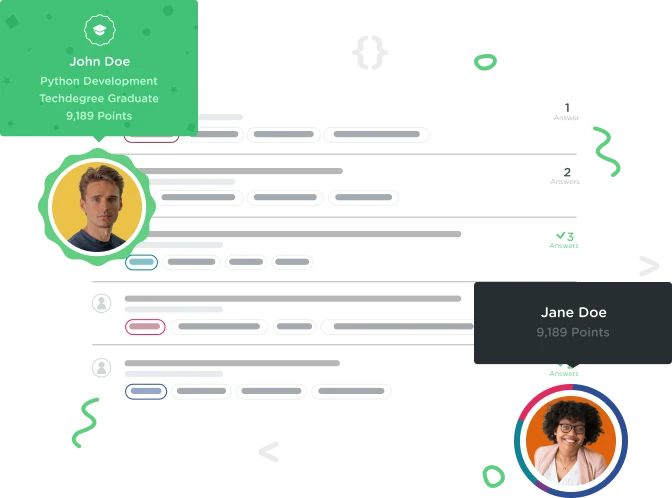

Chris Yan
Full Stack JavaScript Techdegree Student 9,250 PointsDoes an arrow function return a value if there is more than 1 line of code?
Also, what if you don't want the arrow function to return anything (when there's only 1 line of code)?
1 Answer
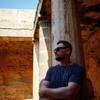
mersadajan
21,306 PointsAn arrow function works very similar to a regular function. It can return a value but it doesn't have to. It depends on what you want to do. You could give it some arguments and let it work on those without returning anything, but you could also give it an object and let it do something with it and let it return that changed object.
The arrow function returning a value is not determined by the length of the code of the function but by the use of the word return
Here is an example:
const a = 1;
const b = 2;
//Here is an arrow function that takes two arguments, adds them together and returns the value of the addition
const arrowFunction = (arg1, arg2) => return arg1 + arg2;
//You can use that function like this. You assign the variable c what the function, arrowFunction, returns when you give it the variables a and b as arguments.
const c = arrowFunction(a,b);
//You could have done that on more lines as well and the functionality and use would be the same.
const arrowFunction = (arg1, arg2) => {
const addition = arg1 + arg2;
return addition;
};
//You can also change the function to not return anything. It would directly save the addition to the variable c without returning anything.
const arrowFunction = (arg1, arg2) => c = arg1 + arg2;
//And you would use it like this:
arrowFunction(a,b);
Hope this helps.