Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial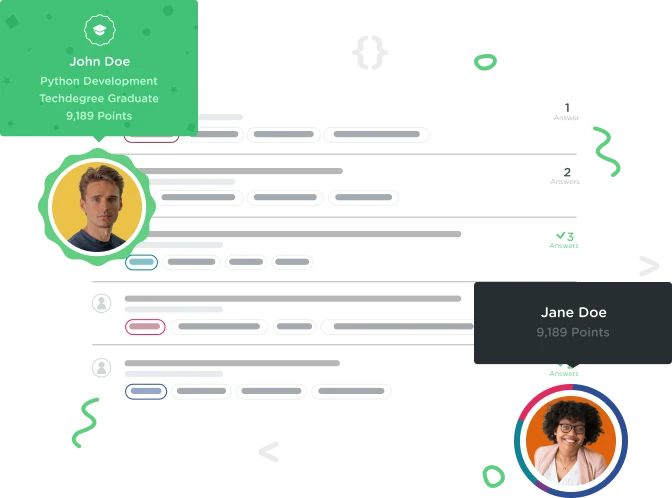

james oliver
Courses Plus Student 684 PointsDoes Anybody knows why when I use a controller in AngularJS I have an error.
<!DOCTYPE html>
<meta charset="UTF-8">
<html ng-app>
<head>
</head>
<body>
<div class="div" data-ng-controller = "simpleController">
<h3>Introduce your name:</h3>
<input type="text"/>
<table>
<tr>
<th>Name</th>
<th>Surname</th>
<th>Age</th>
</tr>
<tr data-ng-repeat = 'customer in customers'>
<td>{{customer.name}}</td>
<td>{{customer.surname}}</td>
<td>{{customer.age}}</td>
</tr>
</table>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.js"></script>
<script>
function simpleController($scope){
$scope.customers = [{name : "mike", surname: "rodrigues", age: 24},
{name : "jose", surname: "ro", age: 23},
{name : "james", surname: "rigues", age: 14}
];
}
</script>
</body>
</html>
The error that im receiving is this: Error: [ng:areq] Argument 'simpleController' is not a function, got undefined.
The thing is that when I want to use a controller I have this error. Can anyone help me to spot what im missing? thanks in advance
2 Answers
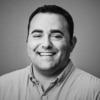
mikes02
Courses Plus Student 16,968 PointsI'll admit AngularJS is not my strongest subject, but glancing through their docs and comparing it to your code I made some adjustments:
<!DOCTYPE html>
<meta charset="UTF-8">
<html ng-app="myApp">
<head>
</head>
<body>
<div class="div" data-ng-controller="simpleController as simple">
<h3>Introduce your name:</h3>
<input type="text"/>
<table>
<tr>
<th>Name</th>
<th>Surname</th>
<th>Age</th>
</tr>
<tr data-ng-repeat="customer in simple.customers">
<td>{{customer.name}}</td>
<td>{{customer.surname}}</td>
<td>{{customer.age}}</td>
</tr>
</table>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.js"></script>
<script>
var myAppModule = angular.module('myApp', []);
myAppModule.controller('simpleController', function()
{
this.customers = [ {name : "mike", surname: "rodrigues", age: 24},
{name : "jose", surname: "ro", age: 23},
{name : "james", surname: "rigues", age: 14}
];
});
</script>
</body>
</html>
One thing is, after ng-controller and ng-repeat remove the spaces and try to use your quotation marks consistently, it makes for cleaner code in my opinion. I used angular.module and assigned it to the myAppModule variable, you can see that myApp also relates to the ng-app code on the HTML element up top.
On the ng-controller I used "as simple" just to make things easier to type out, see: http://toddmotto.com/digging-into-angulars-controller-as-syntax/ for more info.
Then in the repeat, we reference it again in "customer in simple.customers". With this, there were no errors and your data appeared on screen.

james oliver
Courses Plus Student 684 Pointsvery appreciated mike! have a good one!
james oliver
Courses Plus Student 684 Pointsjames oliver
Courses Plus Student 684 Pointsthanks, I have to admit that my code was working using module but I read that we could do it without module and we can use the controller without dot syntax (in this case simple.customers). but might be an update or something. I will use your method from know. thank yo very much for your answer
mikes02
Courses Plus Student 16,968 Pointsmikes02
Courses Plus Student 16,968 PointsYou may be able to, I am not an expert with AngularJS so definitely don't take my word for it completely, this was just me researching their documentation and seeing what worked to get your code to work.