Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial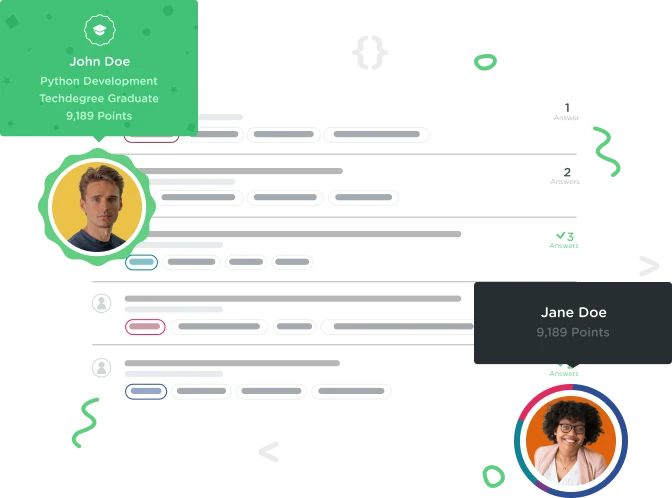

Josip Koprčina
2,690 PointsDoes anybody please know how to solve this
I tried 3/4 things that I tested in visual studio and they work fine there but none work here!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int number = 0;
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
number = int.Parse(Console.ReadLine());
int counter = 0;
while(counter!=number)
{
Console.WriteLine("Yay!");
counter += 1;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
1 Answer
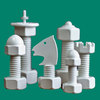
Steven Parker
229,744 PointsThe code you show here passes the first two tasks as is. For task 3, you still need to add code that would check if the input is a negative number and print out the message given in the instructions.
If you're working on task 3, please show the code you're having trouble passing the challenge with. But one hint to start with: be sure you do only what the challenge asks for. One common error that would only show up in the challenge and not external testing is adding extra messages or operational features to the program.
Josip Koprčina
2,690 PointsJosip Koprčina
2,690 PointsThank you ^^ but I was kinda hoping somebody could solve it for me so that i can see what's the best way to solve the problem
Steven Parker
229,744 PointsSteven Parker
229,744 PointsI wouldn't be comfortable "solving it for you", but if you finish task 3, and show your completed code, I'd be happy to give you pointers on possible improvements.
I will say that your task 2 code is very good so far, and the only suggestion I might have at this point is to use a relational comparison ("<") instead of an inequality comparison ("!=") in the loop.
Josip Koprčina
2,690 PointsJosip Koprčina
2,690 Pointsusing System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { int number; int counter = 0;
}
Steven Parker
229,744 PointsSteven Parker
229,744 PointsThat doesn't look like it would pass the challenge, because of a few issues:
Once you fix those issues, I don't see anything else that can be significantly improved.