Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial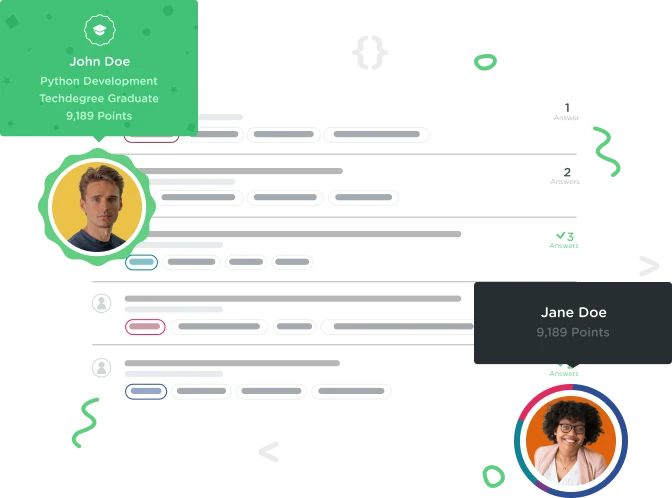

Sandy Woods
1,082 PointsDoes anyone have a step by step approach for the refactoring code challenge?
I"m not quite sure how to even approach this

Sandy Woods
1,082 PointsThe Defaulting Parameters one
5 Answers
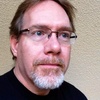
Chris Freeman
Treehouse Moderator 68,441 PointsExample.java
// Task 1 of 1
// So at your new Java job, you've written a brand new Shopping Cart system for the website. After some developers have used the objects you created for a while, they ask you to make it easier to add things to the cart.
// Check out more instructions in Example.java. Once you implement their request, check your work, and you will pass the challenge.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
// Step 1: uncomment line:
cart.addItem(dispenser);
}
}
ShoppingCart.java
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
// Step 2: Add new method
// New Method does *not* have quantity as a parameter
public void addItem(Product item) {
// call the other 'addItem' method specifiying quantity of one:
// adds with default quantity of 1
addItem(item, 1);
}
}
Product.java
// No changes needed to Project.java
Post back if you have more questions
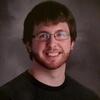
Kevin Michie
6,554 PointsVery well thought out and makes a lot of sense. Thank you for your input!

Sandy Woods
1,082 PointsAlso, as it pertains to the code challenge, I'm never really sure when a statement/ name needs to be passed as a parameter in a method or inside of the body of the method. Can you help me better understand when to pass info in a parameter by using the example above?
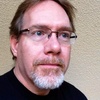
Chris Freeman
Treehouse Moderator 68,441 PointsThe short answer is you need to pass an argument to a function or method whenever the object is not in the current scope or namespace of the method or function. That is, member fields of the class are available to all the class methods and therefore do not need to be passed to those methods.

Sandy Woods
1,082 PointsWonderful Chris. Thanks a bunch. I've gotten quite a bit of helpful info from you. Do you care to give me your e-mail address? I'd like to ask you questions about coding in Java in general and advice as I navigate the uncharted waters (for me ) of doing work in the field.
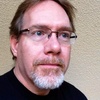
Chris Freeman
Treehouse Moderator 68,441 PointsSorry, I accidentally hit the "mark best answer" button when trying to put this comment in.
Full disclosure: I have much experience in other programming languages but only have picked up Java programming through Treehouse.
It's my other experience in engineering, computer science and Python programming and other languages that has allowed me to help with Java questions.
Questions on succeeding in Java are probably best post to the forum.

Sandy Woods
1,082 PointsThank you

Sandy Woods
1,082 PointsThanks. Also, did you see my previous message about contacting you directly? I wish there was a way I could connect to you and others who have the best answers directly (or maybe there is and I just don't know how/have access to it)
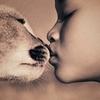
melissakeith
3,766 PointsI tried doing exactly what was done her but it didn't work for me and got many errors
./ShoppingCart.java:8: error: illegal start of expression
public void addItem(Product item) {
^
./ShoppingCart.java:8: error: illegal start of expression
public void addItem(Product item) {
^
./ShoppingCart.java:8: error: ';' expected
public void addItem(Product item) {
^
./ShoppingCart.java:8: error: ';' expected
public void addItem(Product item) {
^
./ShoppingCart.java:8: error: variable item is already defined in method addItem(Product,int)
public void addItem(Product item) {
^
./Example.java:16: error: method addItem in class ShoppingCart cannot be applied to given types;
cart.addItem(dispenser);
^
required: Product,int
found: Product
reason: actual and formal argument lists differ in length
6 errors
Could anyone offer an explanation as to what I'm doing wrong?
[MOD: added markdown formating -cf]
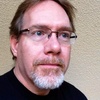
Chris Freeman
Treehouse Moderator 68,441 PointsIt seems you might be missing a semi-colon or a closing brace somewhere for the first addItem
method. It is hard to guess without seeing the code. Perhaps it might be best to post your code in a new forum question if the solutions here don't work for you..
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 Points"Refactoring" is a video. In this section of the course there are three challenges:
Which do you mean?