Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial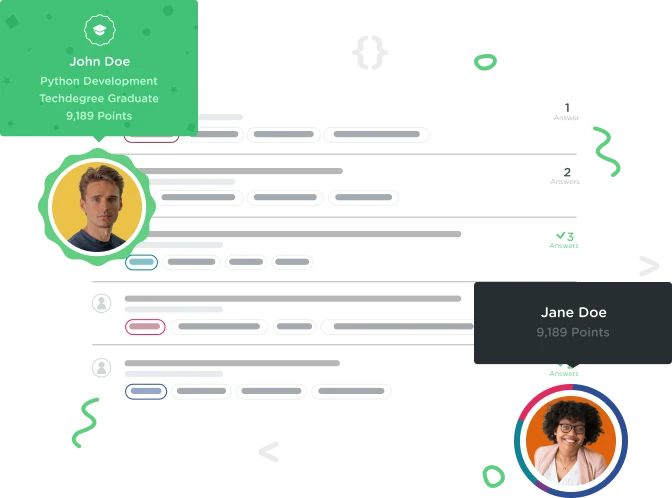
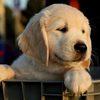
Henry Lin
11,636 PointsDoes anyone know how to write doctest for move_player()?
""" >>> cells = build_cells(3, 3) >>> move_player((1,1), 'RIGHT') (2, 1)
"""
Here is mine but it gave me this:
File "/home/treehouse/workspace/dungeon/dd_game.py", line 83, in dd_game.move_player
Failed example:
move_player((1,1), 'DOWN')
Exception raised:
Traceback (most recent call last):
File "/usr/local/pyenv/versions/3.5.0/lib/python3.5/doctest.py", line 1320, in __run
compileflags, 1), test.globs)
File "<doctest dd_game.move_player[1]>", line 1, in <module>
move_player((1,1), 'DOWN')
File "/home/treehouse/workspace/dungeon/dd_game.py", line 87, in move_player
x, y = player['location']
TypeError: tuple indices must be integers or slices, not str
1 Answer
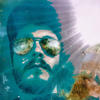
Casey Nord
12,141 PointsThis one confused me for a bit too. What seems to be an obvious answer (and is if we are using the version of this game written in the Python Collections course), turns out to have a few more pieces to it.
If we look back near the beginning of dd_game.py we can see that the variable player is set up like this:
player = {'location': None, 'path': []}
This is how we need to format player when we pass it to the move_player function for testing:
def move_player(player, move):
"""Move player one space in indicated direction
>>> move_player({'location': (1, 1), 'path': []}, 'LEFT')
(0, 1)
"""
x, y = player['location']
player['path'].append((x, y))
if move == 'LEFT':
x -= 1
elif move == 'UP':
y -= 1
elif move == 'RIGHT':
x += 1
elif move == 'DOWN':
y += 1
return x, y
I'm still just starting to gain some momentum with Python, but what I think is happening is that the function loses itself when it tries to unpack player from the dict ['location'], and again when trying to append to ['path'], resulting in the somewhat confusing TypeError.