Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial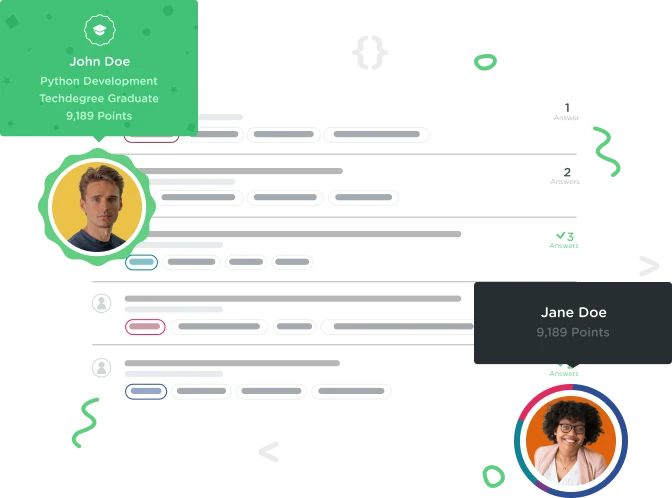

isabelbolger
1,412 PointsDoes not allow me to have calculateArea(12, 10) I must have, width: before the 10. Why is it making me do this?
When Amit calls the functions he simply puts 2 numbers in the parenthesis separated by a comma, however when I try to do it, I get an error and Xcode makes me add width: before the second number. So it would look like this: calculateArea(10, width: 12) or something like that. I am using the latest Xcode not the one used in this video, so is that why this is happening to me?
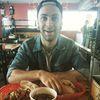
Zaq Dayton
2,244 PointsSo, according to Apple's documentation, when dealing with multiple parameters in a function, all parameters after the first parameter must be labeled. Therefore, here are three examples.
Function with 1 parameter:
var groceryList = ["Apples", "Milk", "Bread", "Eggs"]
func printList(list: String) {
print(list)
}
printList("\(groceryList)")
// Notice that I don't have to use printList(list: "\(groceryList)")
Function with 2 parameters (your example):
func calculateArea(height: Int, width: Int) {
let area = height * width
print("The area of the room is \(area).")
}
calculateArea(12, width: 10)
// Notice that "length:" is not included in front of 12, but "width:" is included in front of "10"
// This is because the first parameter (height) does not need to be labeled, only the parameters after the first one
Function with 3 parameters:
func calculateVolume(length: Int, width: Int, height: Int) {
let volume = length * width * height
print("The volume of the room is \(volume).")
}
calculateVolume(12, width: 10, height: 2)
// Notice that "length:" is not before "12" because it's the first parameter, but the following parameters are "width:" and "height:"
This pattern continues. Therefore, if a function had 10 parameters, the first would not be labeled, but the next 9 parameters would when you called the function. Same thing goes for a function will 1000 parameters, only the 999 parameters following the first would be labeled. Hope this helps!
3 Answers

Michael Benson
674 PointsThe only way that I could get this to work without errors with Swift 2 was to call the first parameter without the label and the second parameter with the label like so:
print("Swift Functions")
func calculateArea(height: Int, width: Int) {
let area = height * width
print ("The area of the room is \(area)")
}
calculateArea(19,width: 10)
On the apple developers site I found the following:
By default, the first parameter omits its external name, and the second and subsequent parameters use their local name as their external name. All parameters must have unique local names. Although itβs possible for multiple parameters to have the same external name, unique external names help make your code more readable.
Link: Function Parameter Names
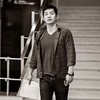
Linh Nguyen
Courses Plus Student 984 PointsChris,
That helped a lot. Thank you!
Christopher Augg
21,223 PointsHello Isabelbolger,
Do you have a signature like:
func calculateArea(height: Int, #width: Int)
When you have a # in front of an argument it will require it during the function call. It is a good practice to do this and you should do it for height as well.
Regards,
Chris

isabelbolger
1,412 PointsThanks!
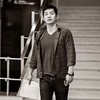
Linh Nguyen
Courses Plus Student 984 Pointsmy Xcode wouldn't let me do this without giving an error. The only way I can make it go away is put height and width in the parameters when I call the function like
calculateArea(height: 1, width: 20)
What should I do?

riyajain23
2,348 PointsWhen I was trying this, it only worked the way Michael Benson did it above. Does Xcode just keep updating this stuff? (Which makes no sense, since that changes the way of programming and might cause a programmer to have to re-write large pieces of code when finalizing an app.)
Christopher Augg
21,223 PointsChristopher Augg
21,223 PointsLinh,
You are correct. Using the # has recently been removed from Swift. The way I see this working now is to do the following:
Notice that you do not have to use two width words. You can also take out the second height, but then when you call calculateArea you would only pass in the number for height while still being required to use width : number. I prefer the former as it is very clear what I am passing to my function.
Please let me know if this helps.
Regards,
Chris