Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial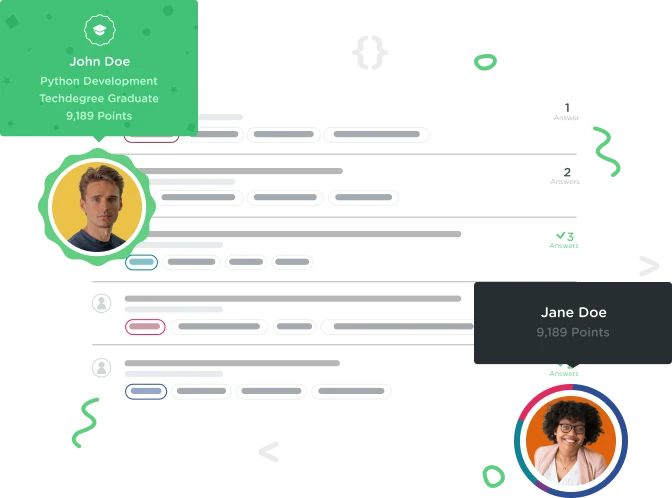

geoff t
Full Stack JavaScript Techdegree Student 1,355 PointsDoes not attach to listDiv but goes in body
seems to go in the body. How can i change this. thanks
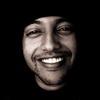
miikis
44,957 PointsWtf. geoff t, is that you down-voting the answers or some trigger-happy moderator?

geoff t
Full Stack JavaScript Techdegree Student 1,355 PointsSorry think that was me by mistake not used the forum part of the site before
now i know what what there for :)
3 Answers
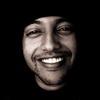
miikis
44,957 PointsHi Geoff,
document.write() doesnโt do what you think it does; in effect, it just replaces whatever is currently loaded in the document. Also, you donโt really need to use a function here โย check out the Array#forEach() API. What you really want to do is this:
var playList = [
['I Did It My Way','Frank Sinatra'],
['Respect','Aretha Franklin'],
['Imagine','John Lennon'],
['Born to Run','Bruce Springsteen'],
['Louie Louie','The Kingsmen'],
['Maybellene','Chuck Berry']
];
// Store a reference to the #listDiv element
var $listDiv = document.getElementById('listDiv'),
// Create an 'ol' element
$ol = document.createElement('ol');
// This is a way easier and readable way of iterating through an array
playList.forEach(function (song, index) {
// for each iteration you:
// create a brand-new 'li' element
var $li = document.createElement('li');
// Add some text to it
$li.textContent = song[0] + ' by ' + song[1];
// And append that 'li' element to that 'ol' element up there
$ol.appendChild($li);
})
// finally you append the 'ol' element to the #listDiv element
$listDiv.appendChild($ol);
Let me know if you have any questions.
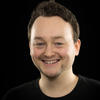
Dom Talbot
7,686 PointsHey Geoff,
If you want to your printSongs function to output to listDiv, you could do something like:
function printSongs( songs ) {
var listDiv = document.querySelector('#listDiv');
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + '| ' + songs[i][1] +'</li>';
}
listHTML += '</ol>';
listDiv.innerHTML = listHTML;
}
<!-- Also make sure to remove the fixed height -->
<div id="listDiv" style="background-color: #ccc;">
<!-- printSongs outputs list here -->
</div>

geoff t
Full Stack JavaScript Techdegree Student 1,355 PointsMany thanks both.

geoff t
Full Stack JavaScript Techdegree Student 1,355 PointsMikis like your answer but abit advanced at moment for me but ill keep it for reference cheers.
can you let me know what the index in the function is used for.
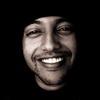
miikis
44,957 Pointsindex is just a parameter; I could've named it anything or just left it out. In fact, I probably should have left it out in this case since I ended up not needing it. In any case, the second parameter of that function will always contain the index of the current element โย in this case, the index of the current song โ being processed. The first parameter of that function will contain the a reference to the current element โ in this case, the actual, current song โ being processed. And the third parameter contains a reference to the original array that all of this shit is happening upon... but I didn't need it here so I didn't include it.
Hope that made sense. Happy learning ;)
You can read more about how the Array#forEach() method works on the MDN site.
geoff t
Full Stack JavaScript Techdegree Student 1,355 Pointsgeoff t
Full Stack JavaScript Techdegree Student 1,355 Pointshttps://w.trhou.se/e4xrgamh7e