Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial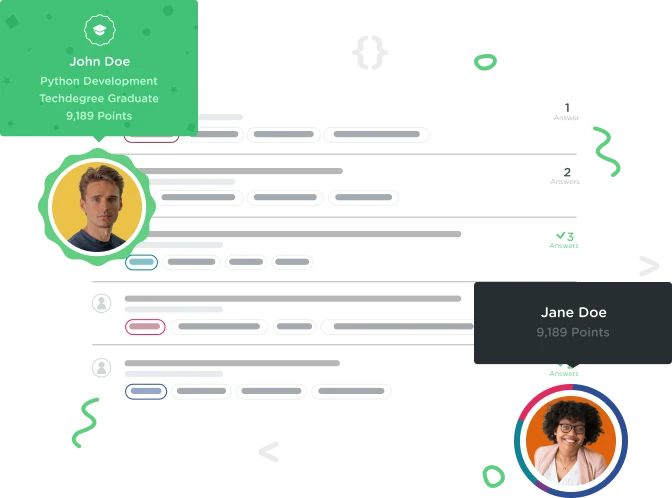

Rujuta Shinde
1,712 Pointsdoes not remove consecutive vowels in the vowel removal function
def disemvowel(word): word_list = list(word) print (word_list) for alpha in word_list: print (alpha) if alpha in ["a","e","i","o","u","A","E","I","O","U"]: #try: word_list.remove(alpha) #except ValueError: #print("alpha {} not found".format(alpha)) print(word_list) else: print("not there") print(word_list) continue i = 0 word1="" while i<len(word_list): word1 += word1.join(word_list[i]) i=i+1 return word1
def main(): w = disemvowel("oeRGo") print (w)
main()
def disemvowel(word):
word_list = list(word)
print (word_list)
for alpha in word_list:
print (alpha)
if alpha in ["a","e","i","o","u","A","E","I","O","U"]:
try:
word_list.remove(alpha)
except ValueError:
print("alpha {} not found".format(alpha))
print(word_list)
else:
print("not there")
i = 0
word1=""
while i<len(word_list):
word1 += word1.join(word_list[i])
i=i+1
return word1
OP:
treehouse:~/workspace$ python vowels.py
['o', 'e', 'R', 'G', 'o']
o
['e', 'R', 'G', 'o']
R
not there
['e', 'R', 'G', 'o']
G
not there
['e', 'R', 'G', 'o']
o
['e', 'R', 'G']
eRG
1 Answer
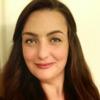
Jennifer Nordell
Treehouse TeacherHi there! Yes, you're correct it will not remove consecutive vowels and there's a reason for this. You're mutating the thing you're iterating over which leads to some unexpected results. The indexing of the iterable is changing every time something is removed. The side-effect of this is that every time a remove
happens, the subsequent letter will not be checked as it has "fallen down" into the place of the thing that just got removed. This isn't a problem when the next letter is a consonant, but obviously, is a problem if it's a vowel.
To mitigate this, you should first create a copy of the iterable. Iterate over the original iterable and use the remove
on the copy. When you're done, rejoin the changed version into a string and return it.
Hope this helps!
Rujuta Shinde
1,712 PointsRujuta Shinde
1,712 Pointsyup that helped! thanks!