Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial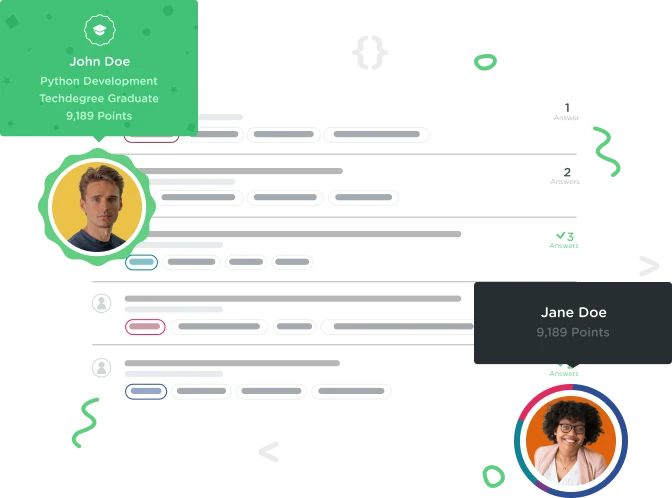
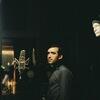
Joseph Michelini
Python Development Techdegree Graduate 18,692 PointsDoes passing an arrow function expression for the first time as an argument to a method mean that I can't use it later?
For example, if I type:
window.setTimeout((something) => {
console.log(something);
}, 3000, 'Greetings Everyone!');
as opposed to:
const greeting = function(something) {
console.log(something);
}
followed by the window.setTimout
code above, with greeting
as the first argument (as opposed to the arrow expression), or even:
const greeting = greeting => {console.log(greeting)}
...there's no way to "quickly" assign my greeting function, correct (referring to the code at the top)? Though I'm led to believe the "best practice" is to avoid declaring variables when you don't have to?
Thanks in advance! Just trying to figure out why & when with all of the above.
2 Answers

Tim Oltman
7,730 PointsHi Joseph,
Passing an anonymous function as an argument means you will not be able to use it later. If you want to use the function later there is nothing wrong with using a function declaration or assigning the function expression to a variable. We use anonymous functions and arrow functions more for convenience and clarity than performance.
Technically, functions will take the value of an assignment expression as an argument. For example, the following code will both assign x the value 4 and pass 4 into the console.log function:
var x;
console.log(x = 2 + 2) // 4
console.log(x) // 4
Therefore, you could assign your arrow function to a variable as you passed it as an argument.
var greeting;
setTimeout(greeting = (something) => {
console.log(something);
}, 3000, 'Greetings Everyone!')
greeting('hi')
In this case you would see "hi" printed to the console followed by "Greetings Everyone!" However, you probably shouldn't do that. Your code will be much clearer if you simply assign or declare your function first.
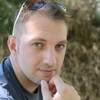
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsHey Joseph! The short answer is no you would not be able to reuse it without declaring the function first. If you wanted to use it again you would need to use something like this:
function say(something){
alert(something);
};
window.setTimeout(say,5000, "shazam!");
say('this happens first');
Here you would get the alert saying "this happens first" followed by the execution five seconds later with the alert saying "shazam!"
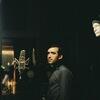
Joseph Michelini
Python Development Techdegree Graduate 18,692 PointsThank you Doron! That makes sense.
Joseph Michelini
Python Development Techdegree Graduate 18,692 PointsJoseph Michelini
Python Development Techdegree Graduate 18,692 PointsThank you! That makes so much sense.