Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial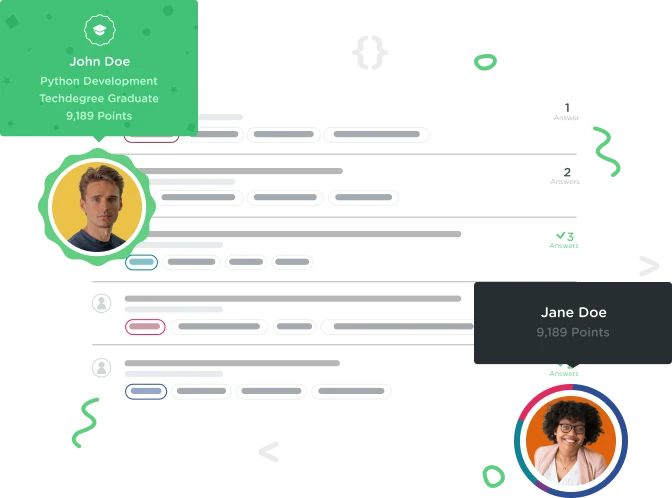
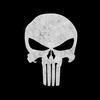
David Ryan
Courses Plus Student 14,981 PointsDoes readyState always have to be set to 4?
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
document.getElementById('ajax').innerHTML = xhr.responseText;
}
};
xhr.open('GET', 'sidebar.html');
function sendAJAX() {
xhr.send();
document.getElementById('load').style.display = "none";
};
2 Answers

cazepeda
6,099 PointsNo it doesn't have to depending on what you WANT to be the readyState from the available values: 0, 1, 2, 3, 4.
Value | State | Description |
---|---|---|
0 |
UNSENT |
Client has been created. open() not called yet. |
1 |
OPENED |
open() has been called. |
2 |
HEADERS_RECEIVED |
send() has been called, and headers and status are available. |
3 |
LOADING |
Downloading; responseText holds partial data. |
4 |
DONE |
The operation is complete. |

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsHi David, the readystate property returns the state the XHR client is in. This property will be updated, when any state changes in your request. The function you've assigned to the onreadystatechange will be called if any of this state-changing events happens.
See https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/readyState
The === operator is a comparison operator and not to "set" something (this would be a single = operator). So actually you are asking if the readystate of the request is the number 4, which means state is "DONE" and the operation is completed. Any state below the number 4 would not make sense, because the request is still pending and not ready for you to work with the data you requested. Any number above 4 would never be in the state and your code would not be triggered.
You'll find more examples for XHR browser API here: https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/Using_XMLHttpRequest
Also more general information about the whole concept and for working with AJAX can you find here: https://developer.mozilla.org/en-US/docs/Web/Guide/AJAX/Getting_Started
Working with asynchronous APIs can be tricky sometimes. Happy coding!
Begana Choi
Courses Plus Student 13,126 PointsBegana Choi
Courses Plus Student 13,126 Pointswow! the table is very helpful. thank you so much!!