Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial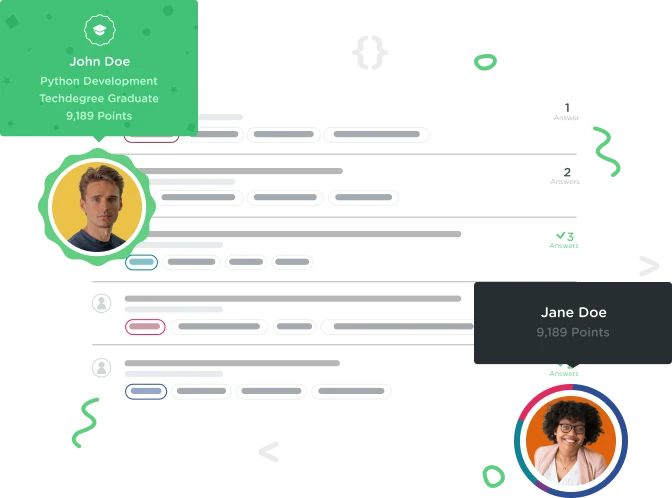
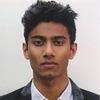
Samuel Kleos
Front End Web Development Techdegree Student 12,780 PointsDoes .resolve() either call a function, resolve to value or accept a value, or is the promise doing these things?
Resolve has a lot of varied uses..
Some places it's not passed anything at all but still 'returns' a promise object. I.e: resolve()
Some places it's supplied a variable as an argument. I.e. resolve(value)
Other places it "returns a response object" or " returns a promise that resolves to a response object" (not sure why the terminology is different or if they mean different things). I.e. resolve(data)
Here's a RESOLVE(value) Example:
const value = 5;
This promise passes value
along with chained .then() methods.
const mathPromise = new Promise( (resolve, reject) => {
setTimeout( () => {
// resolve promise if 'value' is a number; otherwise, reject it
if (typeof value === 'number') { //❓ When is this passed into the promise for evaluation?
resolve(value);
} else {
reject('You must specify a number as the value.')
}
}, 1000);
});
Aside: When the promise below is called, how is the initial value for the value
variable required in addFive passed into the promise in first place?
function addFive(n) {
return n + 5;
}
function double(n) {
return n * 2;
}
function finalValue(nextValue) {
console.log(`The final value is ${nextValue}`);
}
mathPromise
.then(addFive)
.then(double)
.then(finalValue)
.catch( err => console.log(err) )
Here's a RESOLVE(callback) Example:
new Promise( resolve => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload(() => resolve(JSON.parse(xhr.responseText)));
xhr.send();
});
Here's another unique Example where the callback is not in resolve, but in the .then() method!!
fetch(astrosURL)
.then( response => response.json())
1 Answer
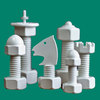
Steven Parker
230,274 PointsBe careful not to confuse the resolve parameter of the function passed when creating a Promise with the .resolve() static method which creates a new promise in an already-resolved state (or resolves and existing promise). For more information on the first, see the MDN page for Promise and for the second, see the MDN page for Promise.resolve().
In the sample code, the value passed to addFive comes from the fulfillment of mathPromise, which directly accesses the global variable value when it calls resolve(value)
in its code. And the answer to "When is this passed into the promise for evaluation?" is when the setTimeout completes, 1 second after mathPromise is created.
And in the final example, there's no access to the creation of the promise returned by fetch, so implementing the callback using .then() is the only option.
Did that answer all the questions?
Samuel Kleos
Front End Web Development Techdegree Student 12,780 PointsSamuel Kleos
Front End Web Development Techdegree Student 12,780 PointsTough gig...
I really appreciate your help. This Unit can be quite a pernicious sticking point without you realising, since there's no practices or challenges. Even the project doesn't require understanding anything about promises. (You just copy-paste the fetch request in the Unit 8 Project).
My approach to getting around this is I'd like to identify the situations Promises can fail without any errors being logged to the console. That way I can see all the stages of promise evaluation by looking at how they go wrong on each stage and try to log them myself.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsPromises fail silently be default. If you want to always display something, make sure to use the "reject" parameter of the callback when creating a promise, and/or chain on a call to the ".catch()" method of an existing Promise.