Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial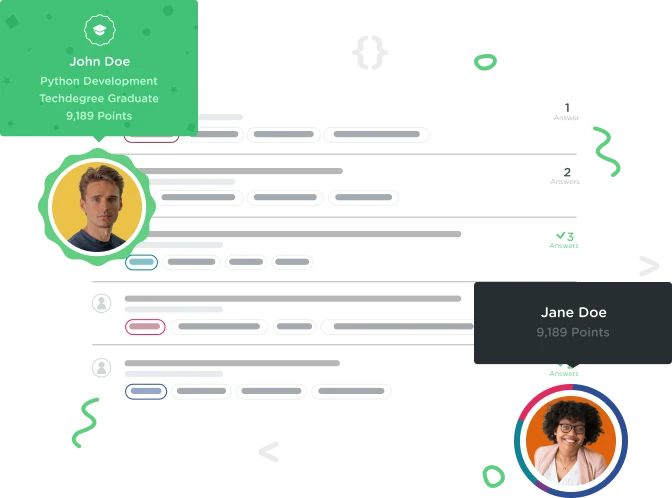

Alex Flores
1,474 PointsDoes the exclamation change the value of CorrectGuess or just check to verify that correctGuess is still false?
I am referring to the condition in the while part of the "do...while" loop. Thanks in advance.
7 Answers

andren
28,558 PointsIt changes what CorrectGuess
evaluates to, but it doesn't change what is actually stored in it. Basically the !
operator takes the result of any Boolean
value and reverses it. So if the value of CorrectGuess
is false
it is treated as true
, and vise versa. But this is not a change that is stored back in the variable.
Also since there appears to be quite a bit of confusion about this, I'll clarify how the while
statement looks at the condition you give it.
When you provide a condition to a while
statement all it cares about is whether that condition evaluates to true
or false
. If it evaluates to true
it runs, if it evaluates to false
it does not run. When you perform a comparison that operation actually results in a Boolean
value that reflects the result. So 5 > 3
becomes true
, 2 === 10
becomes false
and so on. This means that the while
statement does not really look at and care about comparisons, it only looks at the Boolean
value it ultimately receives. This holds true for other conditional statements like if
as well.
That means that when the program starts out (with correctGuess
equal to false
) the !correctGuess
condition evaluates to true
. Since the while
loop only cares about whether it is passed true
or false
this is an acceptable value for it to receive and it runs. Once correctGuess
is set equal to true
within the loop the !correctGuess
condition will now evaluate to false
(since it always becomes the opposite of the Boolean
it is used on) and once the while
statement receives false
as its condition it stops running.
Edit: Added the additional info about how conditions are processed by the while
statement.

Alex Flores
1,474 Pointshmmm thanks. I think I get that in theory, but practically not so much.
Isn't correctGuess already false to begin with? And assuming that the user did not guess the correct number, wouldn't the if statement just be skipped and the condition of correctGuess already being false continue as it was already established?
In that case wouldn't we then just need to verify that correctGuess is still false rather than reverse anything that hasn't even been changed in the first place?
OR is "! correctGuess" just a quick way of saying "If correctGuess is false, that's true, as in the true condition I want this code to be in to continue running" If that's right I'm guessing it's because a while condition won't even run if it's false, so this is a workaround eh?
Sorry for the deluge of questions!

andren
28,558 PointsA while loop (and if statements and the like) will only run when the condition it is given evaluates to true. This means that without the !
reversal the do...while loop would exit after one run even if the answer was wrong (since correctGuess
is not true
), by reversing the value you make it behave in the opposite way.
With !
the loop will treat correctGuess
as true
when it is false
, and false
when it is true
. So your last assumption is more or less correct. You are making the while loop run when correctGuess
is false
, and stop when it is true
. Even though that is the opposite of how loops tend to work.
You don't have to use !
to achieve this, a condition like correctGuess === false
would also work. But that's far wordier than using !
and will look strange to coders used to the !
operator.

Alex Flores
1,474 PointsAh gotcha, thank you so much makes sense now!

Audra Ogilvy
3,142 PointsThat answer still doesn't make sense to me, because if the guess is correct, the correctGuess variable changes to true, which would mean, according to the explanation above, that the while condition would keep the guesses coming when the guess was correct (because if !correctGuess changes that newly changed true value back to false), which would give us the opposite of what we want.
I think your initial question was in the right direction. I think that the exclamation point is just checking to see if correctGuess is false, regardless of what was put in the correctGuess variable. We weren't taught this, though, so I'm not positive. But that's the only thing that makes sense out of this for me.

andren
28,558 PointsThat answer still doesn't make sense to me, because if the guess is correct, the correctGuess variable changes to true, which would mean, according to the explanation above, that the while condition would keep the guesses coming when the guess was correct
No, it would not, because if correctGuess
is true
the the !
mark treats it as false
.
A while
loop does not care about what value is stored in a variable, it only cares about whether the condition you give it evaluates to true
, if it doesn't then the loop ends.
I think that the exclamation point is just checking to see if correctGuess is false, regardless of what was put in the correctGuess variable.
Here is the official explanation of what the ! mark does:
! Logical complement operator, inverts the value of a boolean
The ! operator inverts Booleans
, that's all it does. So true
becomes false
and false
becomes true
.
Since correctGuess
starts out as false
the ! treats it as true
, which makes the while loop run. Then later when you change correctGuess
to true
the ! treats it as false
, which causes the while loop to end, since it will only run while it is given the Boolean
true
.
The effect of that is that the while loop ends up running when correctGuess
is false
, and stops when it is true
. That is not really under dispute, what was being discussed in this thread was the technical details behind how it resulted in that, not the effect that it ultimately had.

Audra Ogilvy
3,142 PointsI think I get it now. Seems like a very confusing way of coding something that could be typed as:
} while (correctGuess === false)
Thanks for taking the time to write. :)

Michael Evans
896 PointsApologies if my question is already answered in the dialogue above. I read all of it and still can't understand why the boolean value of correctGuess needs to be inverted using the "!".
If correctGuess is given a false value to begin with, why can't we write:
if ( parseInt( guess ) === randomNumber ) { correctGuess = true; } } while ( correctGuess )
It seems to me, correctGuess should remain false until the "if" function is met, and not need the "!" since the value has already been designated as false at the beginning. Is a while loop unable to recognize previously established variable values?
Thanks
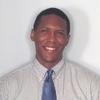
Vaughn Council
2,419 PointsJust wondering why do we need to use the ! operator. I understand what it does as far as changing boolean values to the opposite - but what is the technical reason for writing code using ! vs. correctGuess===false ? Understanding the technical purpose/context might make it easier for everyone to truly understand how to use the operator properly.