Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial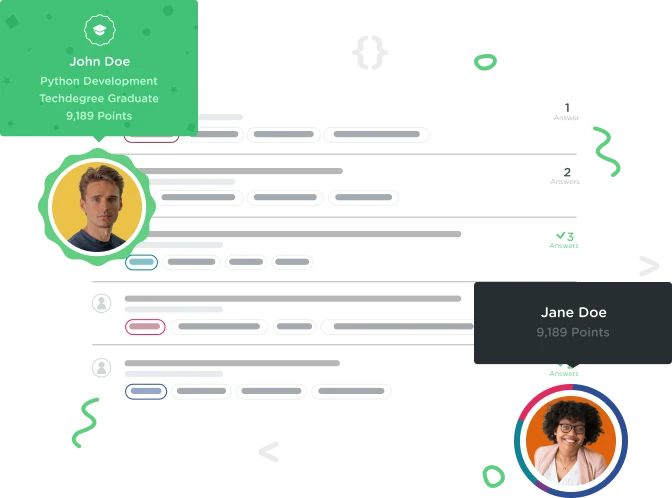
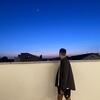
Dominick Chism
8,857 PointsDoes the program she demonstrated make logical sense?
In this program, she attempts to run the go()
method twice by doing:
car_one.go("slow")
car_one.go("fast")
It only runs once however due to the gas running out by the time when it loops through the if self.use_gas():
the second time on car_one.go("fast")
.
My question is how does that make sense not to run? I get why it doesn't, but would this ever be used in a real world scenario? Because there is still 50 in the tank when car_one.go("fast")
is run, but it will run the self.stop()
method instead despite there being 50 in the gas tank still.
Would it make more sense to have if self.gas < 0:
instead of if self.gas <= 0:
? Hope my question makes sense.
Full program:
class Car:
# class attributes
wheels = 4
doors = 2
engine = True
# instance attributes (created with an initializer inside of the class)
def __init__(self, model, year, make="Ford"):
self.make = make
self.model = model
self.year = year
self.gas = 100
self.is_moving = False
def stop(self):
if self.is_moving:
print("The car has stopped")
self.is_moving = False
else:
print("The car has already stopped")
def go(self, speed):
if self.use_gas():
if not self.is_moving:
print("The car starts moving")
self.is_moving = True
print(f"The car is going {speed}")
else:
print("You have run out of gas")
self.stop()
def use_gas(self):
self.gas -= 50
if self.gas < 0:
return False
else:
return True
# instances
car_one = Car("Model T", 1908)
car_two = Car("Phantom", 2020, "Rolls Royce")
# access instance attributes using dot notation (only on an instance, not a class)
car_one.stop()
car_one.go("slow")
car_one.go("fast")
car_one.stop()
4 Answers
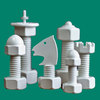
Steven Parker
231,268 PointsI see your point, but I think the original code does make sense. As shown, the car will be "out of gas" and stopped at the end of the move that begins with 50 in the tank. This does fit a real-world model. The only downside is the output did not report that the car was moving before running out of gas.
Perhaps your concerns might be better met with a modification to the "go" method, to report the motion first and then use the gas and test for outage:
def go(self, speed):
if self.gas > 0:
if not self.is_moving:
print("The car starts moving")
self.is_moving = True
print(f"The car is going {speed}")
elif not self.use_gas():
print("You have run out of gas")
self.stop()
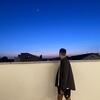
Dominick Chism
8,857 PointsThanks, Steven!

Jennifer Sanchez
8,536 PointsThe issue is, things were not called properly when the instructor gave this code as an example, after playing with it, I realized, first you should use the gas in the method, then call the method and let the method know True or False if self.is_moving. See my code with comments...
class Truck:
wheels = 4
engine = True
doors = 2
# Dunder(double under_score) initializer
#set a default value to and arguemnt (FORD).
def __init__ (self, make, model, year= 1998):
self.make = make #set the attributes
self.model = model
self.year = year
self.gas = 100
self.is_moving = False
def go(self, speed):
self.use_gas() #Use the gas if speeding
if self.use_gas(): #Call the use of gas
self.is_moving = True #let code know vehicle is moving
if not self.is_moving:
print('The truck is going going')
self.is_moving = True
print(f'The truck is going {speed}')
else:
print('You have ran out of gas')
self.stop()
def use_gas(self):
self.gas -= 50
if self.gas <= 0:
return False
else:
return True
def stop(self): #If don't add self, it will throw an error
if self.is_moving == False:
print('The truck has stopped')
self.is_moving = False
else:
print('The truck has already stopped')
truck_one = Truck('Nissan', 'Titan')
truck_two = Truck('Chevy', 'Tahoo', 2023)
truck_one.go('fast')
truck_one.go('faster')

Aaron Elkind
1,393 PointsI think the code wasn't done l in a logical, reasonable, intuitive, progressive thought pattern and the complete lack of explanation going through it, even the first stop/go part was, was absolutely ridiculous. They need to redo this whole section and the main section with either Dennis or Ashley.