Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial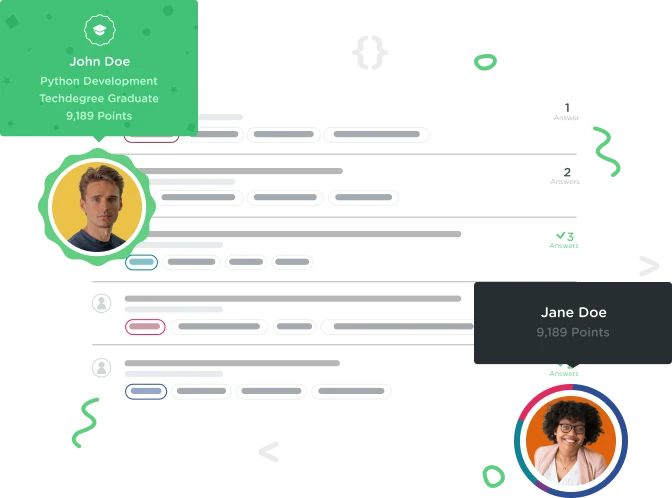

Tim Williams
Courses Plus Student 9,267 PointsDoes the syntax for creating component functions matter?
When creating functions, I normally just put the name of the function followed by its arguments and then the function itself. i noticed that in all the cases for these videos, the name was followed by ": function()". Is there a reason this is required in React? Would this not be properly recognized in some way if i did onStart(){} rather than onStart: function(){}? Code runs fine with no errors but I wanted to confirm if this was style rather than purpose. Apologies if this was covered early on in another video and I happened to miss it.
2 Answers
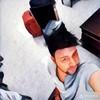
Ari Misha
19,323 PointsHiya there! To be honest , i've been in your shoes actually and i struggled too to wrap my head around different methods to create a component in React. I'll give ya a solution which you can stick to without worrying about literally anything.
Stick to ES6 syntax. Always! Yeah use functional components when you can , otherwise go for class implementation of ES6 syntax. Dont worry about anything else. One important thing that you need to remember while using React is that functional components needs to be pure functions without any side effects. Now, ima show you how to implement React in ES6:
import React from "react"
import { Component } from "react-dom"
class Animal extends Component {
constructor (props) {
super(props);
this.state = {
// .....
}
}
componentWillMount () { ..... }
componentDidMount () { ..... }
render () {
return { };
}
}
export default Animal
This is how you should implement all your components and dont forget about functional components as well. Be sure to use arrow syntax for functional components. I hope it helped! (:
~ Ari

Joseph Wasden
20,407 PointsThe Instructor is using the ":" before function, because he is declaring key/value pairs in an object literal. the value of his key/value pairs are anonymous functions.
{
key: value,
anotherkey: function() {}
}