Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial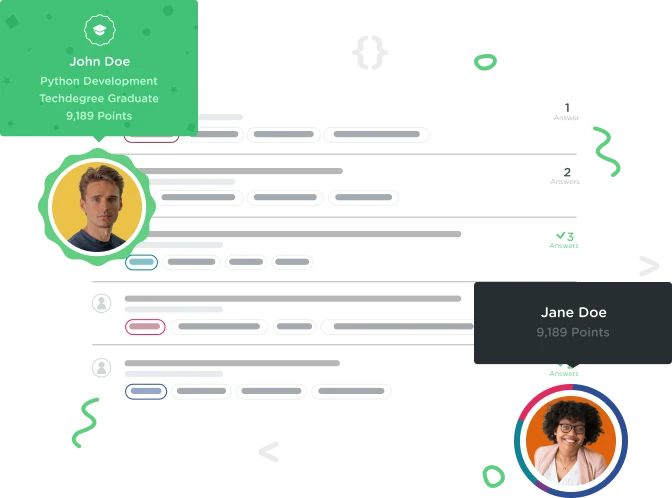
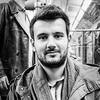
Cristian Glodeanu
4,031 PointsDoes this solution look....Pythonic? :)
Hi,
This is how I solved the code challenge:
def disemvowel(word):
letter_list = list(word)
vowel_list = list("aeiouAEIOU")
for letter in vowel_list:
while (letter in letter_list):
try:
letter_list.remove(letter)
except:
break
word = ''.join(letter_list)
return word
It's a solution that reflects the way my brain works, but I was curious if other people see some flaws in it.
Thanks!
1 Answer
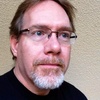
Chris Freeman
Treehouse Moderator 68,441 PointsYes. I would call it "Pythonic". There are always other approaches, but following a natural flow as you see it is often much more readable and understandable than some overly "clever" solution. As for the posted code, I would consider the following changes:
def disemvowel(word):
letter_list = list(word)
vowel_list = "aeiouAEIOU" # This can stay a string.
for letter in vowel_list: # "in" works equally as well on strings and lists
while letter in letter_list: # parens not needed and can be distracting
# since the following command will not execute unless the letter is present
# the try is not needed.
letter_list.remove(letter)
new_word = ''.join(letter_list) # Not good practice to overwrite the argument
# variable as it could affect code outside of the function
return new_word
Beyond being Pythonic, performance is important. Think about how many times the word
list is scanned. The while
scans the letter_list
looking for the current letter
. If one is found, remove()
is called. This also scans the list looking for the letter to remove. So for each vowel that exists, in the original word, the letter_list is scanned twice. As the removing proceeds, all the scans must travel farther and farther down the word to find a match and a removal. This is repeating comparisons that have already been make. (the first not vowel would be compared again on every pass through the loop. It might be better to simply accumulate the "keepers" so it's done in one pass:
def disemvowel(word):
"""10*n comparisons for words of length n"""
result = []
vowel_list = "aeiouAEIOU"
for letter in word:
if letter not in vowel_list:
result.append(letter)
return ''.join(result)
Post back if you have more questions! Keep at it!!
Zach Anderson
3,691 PointsZach Anderson
3,691 PointsI'd be curious if anyone would improve on this as well... that looks similar (but even more concise!) than what I did.