Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial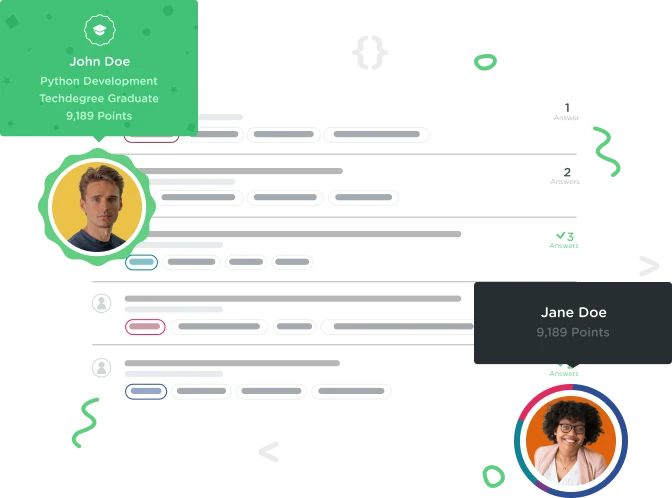

Nicole Freed
695 PointsDoesn't a function need to be initialized/defined before it's called?
In the Functions section, the function "funky_math" is declared before, but initialized/defined after, the "main" function, in which it's called. How does this work? Doesn't a function need to be defined before it's called?
6 Answers

Nicole Freed
695 PointsHa! So I just got the answer from a senior programmer. It has to do with the difference between interpreted and compiled languages. JavaScript is an interpreted language: the interpreter runs and executes the program sequentially, therefore, functions have to be defined before they're called. However, C-based languages are compiled, which means that they're essentially run behind the scenes. C compilers make three passes: in the first, they check to see that all the syntax is correct; in the second, they "connect all the dots"—put together function declarations with definitions, etc.; and in the third, they actually run the program. Therefore, if things are out of order, they're put together in the compiling process, since the compiler doesn't actually execute the program linearly.

Joseph Taralson
1,737 PointsAndrew - here's the best way to think about it:
Yes, you are correct that you can code the way you did in your example and it will work fine.
However, when coding a larger program, organizationally this is impractical. Your main() function is the body of your program, and in a larger program you may have 10, 20, or 100 additional functions you reference in the main() function. The reason we are taught to first declare functions above main() and then define after main() is for organization. In a large program you would have to scroll through lines and lines and lines of functions before getting to your main() function, which tells you what the heck all those other functions are doing.
Make sense?
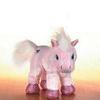
Andrew King
3,411 PointsI'd forgotten about this thread! Yeah it does make sense & I came to that same thinking as I worked ahead in the course.
Many thanks for taking the time to reply.
A
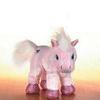
Andrew King
3,411 PointsWhat confused me about this is that the code below runs and produces the exact same output as the version where you declare the function first & then implement it after. Can anyone explain then the need to declare it first and then implement it later? Seems like a wasted line of code that doesn't seem to be doing anything? Or am I missing a bigger picture for a larger scale program?
#include <stdio.h>
int funky_math(int a, int b) {
return a + b + 343;
}
int main()
{
int foo = 24;
int bar = 35;
printf("funky math %d\n", funky_math(foo, bar));
return 0;
}

Nicole Freed
695 PointsIf the code above produces the same output as the code example in the video, it makes me wonder if the C compiler searches through the entire program for the definition of the function before attempting to execute it, rather than executing the program sequentially? If it executes the program sequentially, the code in the video doesn't make any sense (but yours does, Andrew), whereas if it searches for the definition of the function when it encounters it being called, and then executes it, both of the examples would work.

Nicole Freed
695 PointsYou're asking about the reason behind the separation of declaration and implementation, correct, Andrew? I've wondered about that, too. I've read that it's generally better to do both at once, but I can imagine there might be circumstances under which one would want to separate the declaration and implementation (but being a baby beginner in this stuff, I have no idea what those would be).
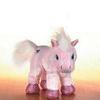
Andrew King
3,411 PointsI came to C from a bit of Java Script background and this is how you do it there. You declare functions & then call them. It just seems like a massive waste to declare a function but not actually include what it 'does'. To then rewrite the declaration THEN include it's initialisation code.
My initial assumption is that on a larger scale program the need to declare it separately is required or if it's simply that the description in the video wasn't too stellar and / or made a mistake.
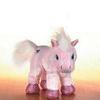
Andrew King
3,411 PointsSo surely by that reasoning it is pointless writing the function the way it's done in the video and you can just write it in one go like I did in the example above. If the compiler will figure it all out, it shouldn't be a problem, no?
You should ask the guy you just spoke to that.

Nicole Freed
695 PointsI might, although it's not my primary concern. I'm sure there are times when one would want to separate declaration and initialization. However, it's your question; why don't you go ahead and put it in a separate thread?
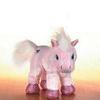
Andrew King
3,411 PointsMy initial statement / question is just the next logical step in the question you initially asked. The answer you received from the person you spoke to simply raises the "yeah, so what difference does it make how it's written?". Seems highly inefficient to make a second fresh thread on the same issue, especially when a discussion is already in motion.
Hopefully one of the Treehouse tutors will see the thread & dive in with a follow up.

Tristan Gaebler
6,204 PointsYour defining the function 'funky_math' before the main function, and manipulating the function after the main function.

Nicole Freed
695 PointsI used the wrong terminology previously; I've updated the question to be clearer. The "funky_math" function is declared before the "main" function, but initialized/defined after the "main" function. However, it's used in the "main" function, before it's defined. How does this make sense?
Robert Mylne
13,708 PointsRobert Mylne
13,708 PointsNicole Freed "JavaScript is an interpreted language: the interpreter runs and executes the program sequentially, therefore, functions have to be defined before they're called."
Then explain why that works.
Alex Sell
19,355 PointsAlex Sell
19,355 PointsYou can read about functional hoisting in JavaScript here: http://adripofjavascript.com/blog/drips/variable-and-function-hoisting