Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial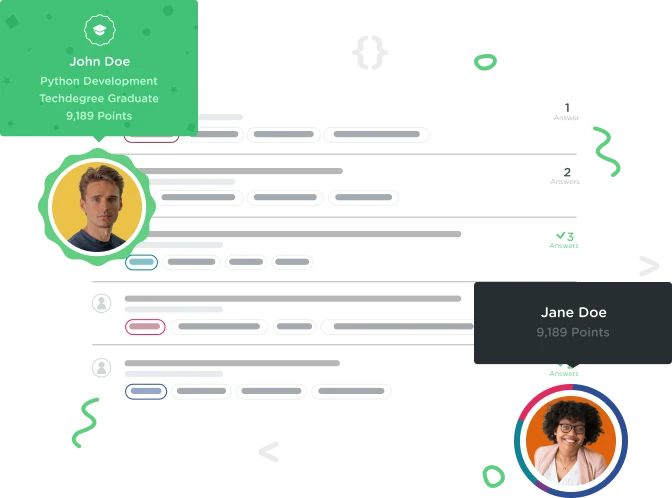
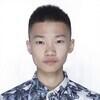
jack twist
Full Stack JavaScript Techdegree Student 18,575 Pointsdoesn't /*array.push(forEach loop)*/ work?
i don't understand why my code doesn't work
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
times5.push(numbers.forEach( num => num*5 ));
1 Answer
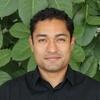
Juan Luna Ramirez
9,038 PointsforEach runs the given function for each item in the array. After forEach is done, it actually returns undefined
. I'll expand on this undefined
return value later to attempt to explain what is actually happening in your code.
This is how you would use forEach
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// again, we need forEach so we can run a function for each item
// to possibly do something with that item. You're not obligated
// to use the item, but generally you do need it like in this case
// where you want to multiple each one by 5
numbers.forEach(num => {
// this is the function we execute for each num. Let's do it
// for demonstration purposes we'll multiple by 5 and save
// that value to a variable
const numberMultipliedBy5 = num * 5
// now we need to put our new number in the times5 array with
// the push method
times5.push(numberMultipliedBy5)
})
that's it times5
should now be populated with the numbers multiplied by 5.
This is a condensed version but essentially it is the same as above
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
numbers.forEach(num => times5.push(num * 5))
Now let's look at what is happening in you code. I'll expand it to hopefully make it clear why it doesn't work
this doesn't work!!!
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// I'm saving the return value of executing forEach
// which is undefined. It's important to note that this
// is not the return value of the function passed to forEach
const thisValueisUndefined = numbers.forEach( num => {
// again this is the function we execute for each num
// we are indeed multiplying each number by 5 but then we
// aren't saving it anywhere. forEach is flexible
// that way but it's your responsibility to save the values
// somewhere if that's what you want. Returning it doesn't
// do anything for us.
return num*5
})
// now we push the return value of running the forEach function,
// NOT the return value from the function passed to forEach. Since
// this value is undefined the times5 array is simply [undefined]
times5.push(thisValueisUndefined);
For reference you can view the mdn documentation for the return value of the forEach function https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach#return_value
As a side note, there are other Array function like map
that will automatically put the newly modified item in an array that is returned after running the map function and you can also see some examples and how the return value of running this Array function is different from forEach https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map#return_value
jack twist
Full Stack JavaScript Techdegree Student 18,575 Pointsjack twist
Full Stack JavaScript Techdegree Student 18,575 PointsThanks a lot! It's very clear, BTW I'm wondering if you are a teacher in treehouse?
Juan Luna Ramirez
9,038 PointsJuan Luna Ramirez
9,038 PointsI'm glad it helped.
I'm not a teacher. I had a treehouse subscription years ago and liked this forum so I try to answer questions when I can. I have an account but my subscription has been cancelled for a long time. Good thing I can still interact with people here! I can't see the challenges or video, obviously, so sometimes I don't know what people are referring to in their questions. haha!
jack twist
Full Stack JavaScript Techdegree Student 18,575 Pointsjack twist
Full Stack JavaScript Techdegree Student 18,575 PointsWow, that's very nice of you! The reason I asked is that the explanation you gave is very clear and easy to follow for me, it's hard to find this kind ;D
Juan Luna Ramirez
9,038 PointsJuan Luna Ramirez
9,038 PointsI appreciate you comments! :) Good luck with everything!