Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial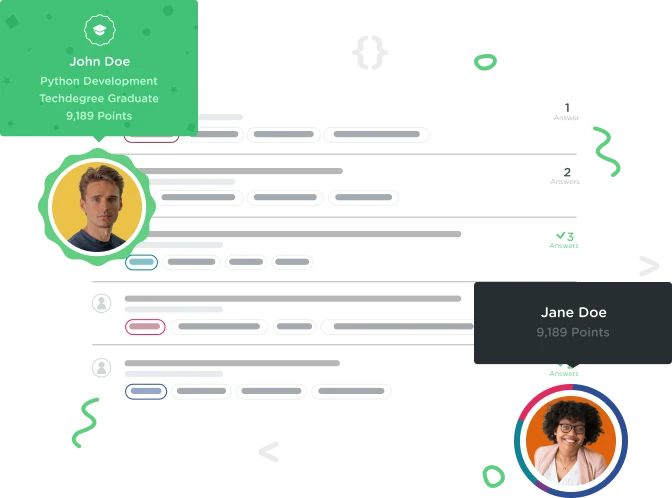
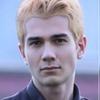
Dinu Comendant
6,049 PointsDoesn't save in Local Storage
For some reason the items the information is not saved in the Local Storage. I tried to track where the problem could be and it seems that the getInvitees works, but saveInvitees does not.
const form = document.getElementById('registrar');
const input = form.firstElementChild;
const ul = document.getElementById('invitedList');
const lis = ul.children;
const myStorage = window.localStorage;
const mainDiv = document.querySelector('.main');
const newDiv = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckbox = document.createElement('input');
const clearUl = document.getElementById('clearStorage');
const clearLi = document.createElement('li');
clearUl.appendChild(clearLi);
const clearButton = document.createElement('button');
clearButton.textContent = 'Clear Storage';
clearLi.appendChild(clearButton);
filterLabel.textContent = 'People who haven not responded';
filterCheckbox.type = 'checkbox';
newDiv.appendChild(filterLabel);
newDiv.appendChild(filterCheckbox);
mainDiv.insertBefore(newDiv, ul);
filterCheckbox.addEventListener('change', (e) => {
const isChecked = e.target.checked;
if(isChecked) {
for(let i = 0; i < lis.length; i++) {
if(lis[i].className === 'responded') {
const respondedLabel = lis[i].childNodes[1];
lis[i].style.display = '';
respondedLabel.style.display = 'none';
} else {
lis[i].style.display = 'none';
}
}
} else {
for(let i = 0; i < lis.length; i++) {
const respondedLabel = lis[i].childNodes[1];
lis[i].style.display = '';
respondedLabel.style.display = '';
}
}
});
function createLi (text) {
function createElement (elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendLi(elementName, property, value) {
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
const li = document.createElement('li');
const p = document.createElement('p');
appendLi('span', 'textContent', text);
appendLi('label', 'textContent', 'Not Confirmed')
.appendChild(appendLi('input', 'type', 'checkbox'));
appendLi('button', 'textContent', 'Edit');
appendLi('button', 'textContent', 'Remove');
return li;
}
form.addEventListener ('submit', (e) => {
e.preventDefault();
//allocate the invitees in a new variable
const pastInvitees = getInvitees();
const text = input.value;
if(text === ''){
alert('There is no name');
}
input.value = '';
const li = createLi(text);
ul.appendChild(li);
});
ul.addEventListener ('change', (e) => {
const checkbox = e.target;
const checked = checkbox.checked;
const listItems = checkbox.parentNode.parentNode;
confirmLabel = checkbox.parentNode.firstChild;
if(checked) {
listItems.className = 'responded';
confirmLabel.textContent = 'Confirmed';
} else {
listItems.className = '';
confirmLabel.textContent = 'Not Confirmed';
}
});
ul.addEventListener ('click', (e) => {
if(e.target.tagName == 'BUTTON') {
const button = e.target;
const li = e.target.parentNode;
const action = button.textContent.toLowerCase();
const nameAction = {
remove: () => {
ul.removeChild(li);
},
edit: () => {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input,span);
li.removeChild(span);
button.textContent = 'Save';
},
save: () => {
const newSpan = document.createElement('span');
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
}
};
nameAction[action]();
}
});
clearButton.addEventListener('click', () => {
removeInvitees();
});
///////////////////LOCAL STORAGE
function supportsLocalStorage() {
console.log('supports');
return ('localStorage' in window) && (window['localStorage'] !== null);
}
function getInvitees() {
console.log('gets');
const invitees = localStorage.getItem('invitees');
if(invitees) {
return JSON.parse(invitees);
} else {
return [];
}
}
function saveInvitees(str) {
const invitees = getInvitees();
invitees.push(str);
localStorage.setItem('invitees', JSON.stringify(invitees));
console.log('adds');
}
function removeInvitees(str) {
const registrants = getInvitees()
const toBeRemovedIdx = registrants.indexOf(str)
registrants.splice(toBeRemovedIdx,1)
localStorage.setItem('registrants', JSON.stringify(registrants))
console.log('remove')
}
window.onload = function () {
if(supportsLocalStorage) {
//remove the invitees
const pastInvitees = getInvitees();
input.value = '';
//add invitees to the local storage
for(i = 0; i < pastInvitees.length; i++){
const newLi = createLi(pastInvitees[i]);
ul.appendChild(newLi);
}
}
console.log('works');
}