Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial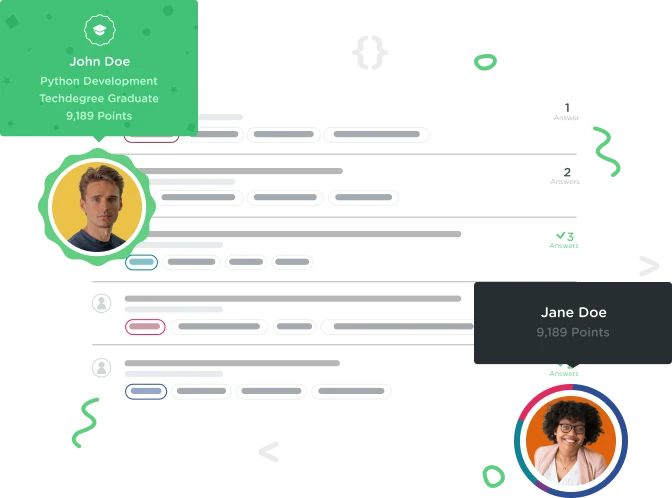
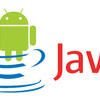
Gábor Tóth
11,958 PointsDoesn't show the ungessed letters as dashes
Hi! I followed every step, and I don't get any compiler errors in the console, I can run my code, but it still doesn't show the ungessed letters as dashes, right from the start. Thank you for your help. Here's my code: Game.java:
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game (String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
private char validateGuess(char letter) {
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess (String letters) {
if (letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
return applyGuess(char letter)
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0); {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
public String getAnswer {
return mAnswer;
}
public boolean isSolved() {
return getCurrentProgress().indexOf('-') == -1;
}
}
Prompter.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while(mGame.getRemainingTries() > 0 && !mGame.isSolved()) {
displayProgress();
promptForGuess();
}
if (mGame.isSolved) {
System.out.printf("Congratulations you won with %d tries remaining\n",
mGame.getRemainingTries());
} else {
System.out.printf("Bummer the word was %s. :(\n",
mGame.getAnswer())
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (!isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
1 Answer

christopherkaczenski
16,079 PointsThere is a stray semicolon in getCurrentProgress() method of the Game class, which is the method that builds the string with dashes. Take a close look at the if statement.