Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial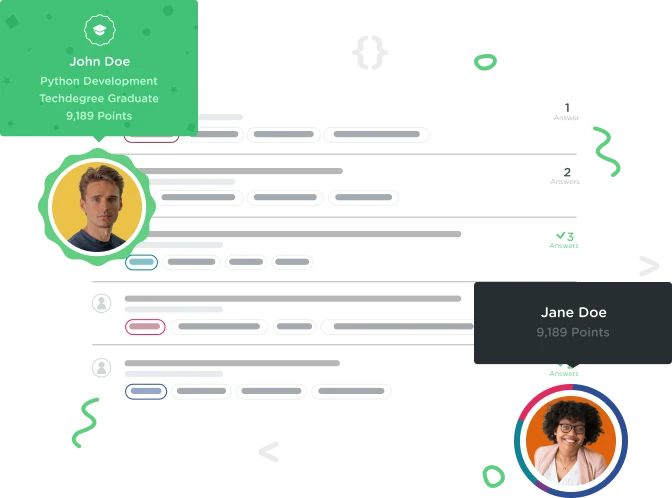
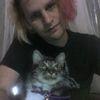
Bryan Wiedeman
10,203 PointsDoesn't suggest "surround with try/catch"
I get to the point where it is erroring on my call.execute() and it is in fact erroring, but it isn't offering me "surround with try catch" as a quick-fix. Neither is try/catch an option when i got into the menu>code>surround. The quick-fixes it offers are
Add method contract to 'execute' Annotate method 'execute' as @Deprecated Annotate method 'execute' as @NotNull Annotate method 'execute' as @Nullable Move initializer to constructor Split into declaration and initialization Convert to ThreadLocal Make 'private' Make 'protected' Make 'public'
my code is as follows
package com.example.paxie.stormy;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
String apiKey = "1621390f8c36997cb1904914b726df52";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
Response response = call.execute();
}
1 Answer
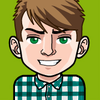
Harry James
14,780 PointsHey Bryan!
Not sure what happened with the date here - sorry if you have been waiting 29 days ago for a response, I seem to have just gotten this ask to answer in my email today...
Anyhow, you seem to have written your code outside of the onCreate() method. All of your client code should be written inside here so that it can be executed.
I've saved you the hassle and put the correct code below - feel free to swap out your code with this:
package com.example.paxie.stormy;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "1621390f8c36997cb1904914b726df52";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
Response response = call.execute();
}
}
As you can see, I have moved this code within the closing curly brace (}
) for the onCreate() method.
All of the code in onCreate() is ran when the activity is created - this is the starting point for all of your code. The only time we write any code outside of this method is for refactoring (If we want to put some code in a separate method to make code cleaner and stop repeating ourselves) or for scopes (If we want to access a variable in more than one method, for example).
With this code in its correct place, you should now see the prompt to surround in try/catch block, give it a go ;)
Hopefully this should explain this for you but if there's anything you don't quite understand, give me a shout :)
Neeru Nayak
1,567 PointsNeeru Nayak
1,567 PointsThanks Harry ..it helped me today :)