Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial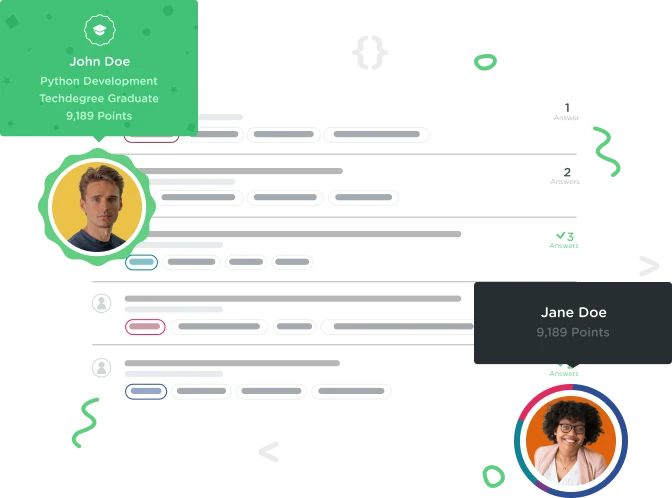

Tony K
Courses Plus Student 442 PointsDoesn't understand the " Exceptions " Video clearly ?
Why is " throw , new, try ,catch "things used here .. can someone plz help me with this !!! having a little bit of problem he...re !
1 Answer

Daniel Hartin
18,106 PointsHi Tony
I didn't really get this example as you could have easily overridden the entered value if bigger than the maximum but the concept of try catch is common among a range of languages and is really useful.
Think of it like this, you design your console app which asks the user for a number, if they type a number and you try to parse a number from the returned value it works! yeah !! and everything is happy. But what happens if you get a user who wants to try and break your code and enters "one" or "hello" if you try to parse a number from these values you would get an unhandled exception (This is like where if you have ever had a phone app and it says The app has crashed please send an error report).
How you get around these unknown's is to first TRY the code to see if it passes but if it does crash you can CATCH the error before it closes your application and you can display a message of your own to the user. Allowing you to ask them again for another input or exiting gracefully (and saving their progress etc etc in the meantime)
The example here shows you how to throw your own exceptions which is a little more advanced, I do use them, but quite rarely if I'm honest in my own code so I wouldn't get too hung up on how to create your own it's a Google search when and if you ever need to use them for yourself but they are littered through most Classes in Java. You should really get used to using try/catch as you will come across it often. Basically whenever you are doing something where there is a possibility that the value may not be the correct type and/or in the correct range use try/catch (especially user input!!!)
You can also catch all using
try{
}catch(Exception e){
}
should you want to, although there are a few reasons why you should use this sparingly which I won't get you lost in right now!
Hope this helps
Daniel
Tony K
Courses Plus Student 442 PointsTony K
Courses Plus Student 442 PointsThank You Sir..but sir can you be more specific by giving me an example to grasp this catch/try method clearly !!
Daniel Hartin
18,106 PointsDaniel Hartin
18,106 PointsHi Tony
Sure, the easiest way to explain this using methods you've probably already used is shown below, the code shows a couple of simple lines where you prompt the user to enter their name and then their age. Now you are fully expecting them to enter data like "Steve" and 25 which (run the code to test yourself in console) if they enter those values works just fine.
You as the programmer are aware that the call to readline always returns a string, but you need the age to be an integer so you parse straight off of the returned value which is fine right? it works.... Well no, not if the user enters something unexpected such as the string "twenty-five", if they enter this you will see something like:
You should be aware of these possibilities when writing code and the best way to prevent these errors where your code exits/crashes is to use a try/catch block to try the code and catch any error that may occur.
The code above wraps the statements that 'MIGHT' cause a crash inside the try block, if any of the code inside the block results in an error the program will check to see if the exception thrown matches any of your exception types, if it does it will run the code inside that block without crashing and allow you to make changes in your code to accommodate.
If you run the script above and when prompted for your age enter the string thirty you will see the print out "No way am I gonna crash!!! enter an integer value next time" this is because trying to parse an integer from a string that doesn't solely contain a numeric value (the string representation of one) it results in an error (to be exact a NumberFormatException)
In short, anywhere where your code could obtain a value that you aren't in full control over use a try/catch block and write code that plans for every eventuality, always think to yourself "will this cause my program to crash" if the answer is even the remotest yes then use try/catch and plan for every eventuality
Hope this helps
Daniel