Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial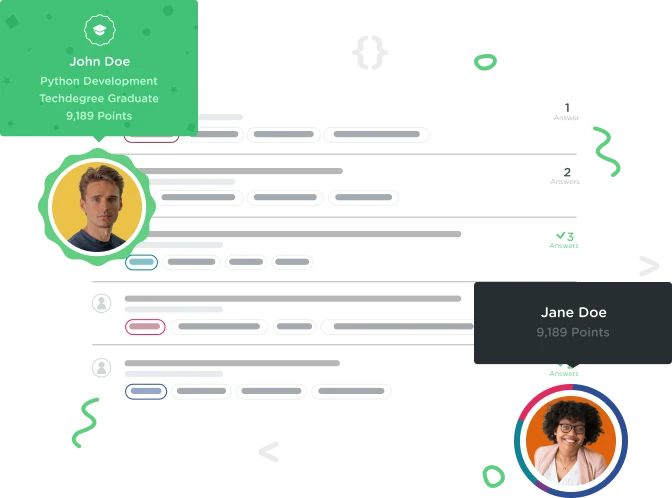

LESTER POLICARPIO
2,139 PointsDoing a test regarding Inheritance which throws error
Hi,
I am using below code to further test the inheritance topic. All I want is to get the value of the method CheckValue() by using the secondary class Square using inheritance instead of the Polygon class. The problem is that it is throwing an error "the name 'numSides' does not exist in the current context".
using System;
namespace Test_On_Inheritance
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
public int CheckValue()
{
return NumSides;
}
public static void Main()
{
Polygon result = new Polygon(4);
Console.WriteLine(result.CheckValue());
Square result2 = new Square(5);
Console.WriteLine(result2.CheckValue());
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(numSides)
{
SideLength = sideLength;
}
}
}
4 Answers
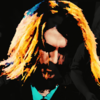
Justin Horner
Treehouse Guest TeacherHello Lester,
The reason you're seeing that error is because the Square constructor is passing numSides to the base constructor, which does not existing in the scope of the Square class.
I hope this helps.

LESTER POLICARPIO
2,139 PointsThanks Justin,
Isn't below part of doing an inheritance?
"public Square(int sideLength) : base(numSides)"
because base on the video tutorial it was done using this "public MapLocation(int x, int y) : base(x,y)" which also was not in the scope of the MapLocation class?
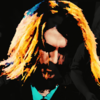
Justin Horner
Treehouse Guest TeacherYes, passing constructor information to the base constructor is a part of inheritance but in this case the compiler is looking for a variable named numSides within the context of Square but it doesn't exist.
You could add a numSides parameter to the Square constructor and then pass it via base. You can also simply pass a number. I've demonstrated these options below.
public Square(int sideLength, int numSides) : base(numSides)
{
SideLength = sideLength;
}
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
I hope this helps.

LESTER POLICARPIO
2,139 Pointsbelow are the code for the MapLocation class which only calls the Point class x and y using base (x,y) and did nothing else in this class.
namespace TreehouseDefense { class MapLocation : point {
public MapLocation(int , int y) : base(x,y)
{
}
}
}

LESTER POLICARPIO
2,139 PointsThanks Justin I got it now =)
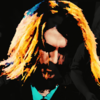
Justin Horner
Treehouse Guest TeacherYou're welcome! Happy coding :)