Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial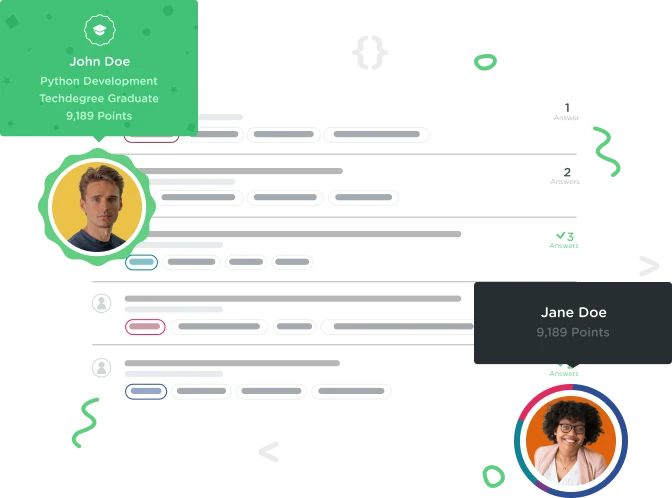

Vic Mercier
3,276 Pointsdom
const myHeading = document.getElementById("vic"); myHeading.addEventListener("click",()=>{
//I don't understand this arrow function and why we put , and why we don't do myHeading=()=>{
} });
2 Answers

Aaron Price
5,974 Pointsconst myHeading = document.getElementById("vic");
// This creates an event listener. Every time it 'hears' a click, the callback function is invoked
myHeading.addEventListener("click", () => {/* callback function goes here */});
// But if you did this instead...
myHeading = () => {/* some function goes here */}
// ... then you would simply reassign myHeading as a function.
Keep in mind that myHeading is an object, and .addEventListener() is a method on the myHeading object. If you don't call the .addEventListener() method, then you don't create an event listener.
It takes two arguments. The first is the event it should listen to; "click", or "scroll", or whatever. The second argument is the function for it to call whenever that event happens.
The syntax is addEventListener(event, callback)
Note also that it doesn't HAVE TO be an arrow function. You could use the old school syntax, or even use a named function. All of these are valid.
function myCallback() {/* callback function goes here */}
myHeading.addEventListener("click", myCallback);
// or
myHeading.addEventListener("click", function() {/* callback function goes here */});
// or
myHeading.addEventListener("click", () => {/* callback function goes here */});

Christopher Debove
Courses Plus Student 18,373 PointsBefore answering your question, we gonna take a look a this code without arrow function.
const myHeading = document.getElementById("vic");
myHeading.addEventListener("click", function() {
// body of event handler
});
You can use arrow function to shorten the basic function syntax. Because their declaration are shorter.
const myHeading = document.getElementById("vic");
myHeading.addEventListener("click", () => {
// body of event handler
});
Also why we don't do myHeading = () => {}
.
If you read my first code you already have your answer. It's because myHeading is not a function. The function is here to be used as a callback in the click event.
Vic Mercier
3,276 PointsVic Mercier
3,276 PointsYour answer was very helpfull and clear!?