Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial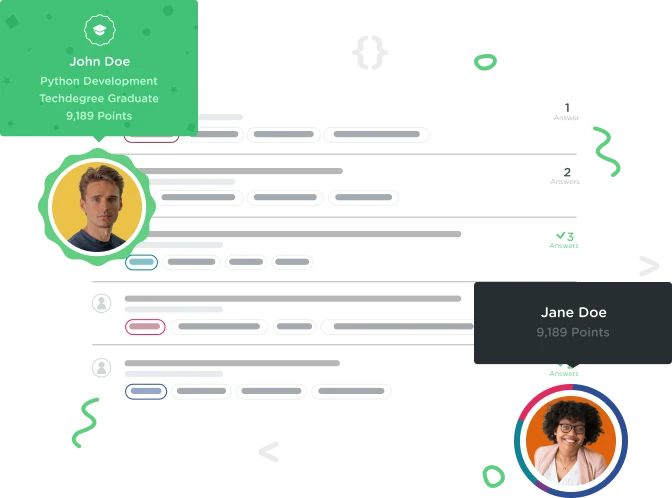

Tim Brooks
2,339 PointsDOM: Can't get list items to turn to rainbow colors
Hi, I'm stuck on getting this code to turn the quiz example to rainbow colors:
var listItems; var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = getElementByID("rainbow").colors(i);
}
var listItems;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = getElementByID("rainbow").colors(i);
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
3 Answers
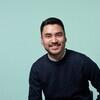
Rabin Gharti Magar
Front End Web Development Techdegree Graduate 20,926 PointsHey Tim Brooks,
First, you need to select <ul>
element with the id
of rainbow
and use the parentNode.children
property.
It should look like this:
var listItems = document.querySelector('#rainbow').children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
To learn about this property, check out this website: https://developer.mozilla.org/en-US/docs/Web/API/ParentNode/children#Syntax
Hope this helps!
Happy Coding!

Tim Brooks
2,339 PointsThanks, that worked! I'm still puzzling over it bit, but hopefully this stuff will sink in.
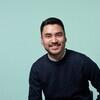
Rabin Gharti Magar
Front End Web Development Techdegree Graduate 20,926 PointsI will break this down into small sections so you can have a better understanding:
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
- Inside
<ul>
there are 7<li> tags
. -
<li>
is thechildren
of<ul>
tag. -
<ul>
has theid
of#rainbow
.
var listItems = document.querySelector('#rainbow').children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
Here in JS, I have selected
<ul>
tag with itsid
using aquerySelector
method and I used achildren property
, this property returns a collection of an element's child elements. In this case, it'll return<ul>
child elements<li>
. I then stored it in avariable
namedlistItems
.Secondly, I created an
array variable
namedcolors
and stored7
different colors which I will apply in<li> tags
stored inside<ul>
.And finally, I used a
for loops
to iterate through each<li>
tags and apply the color to each of them. A for loop repeats until a specified condition evaluates to false.
Hope this helps!
Happy Coding!