Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial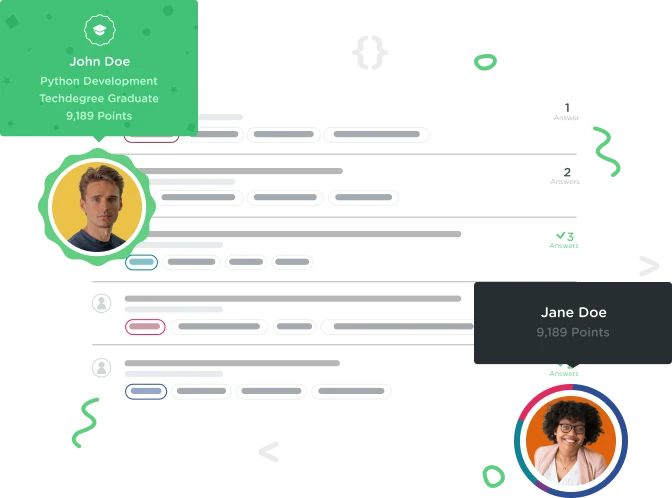

Seth wright
952 PointsDOM rendering in separate file on Visual Studio... how can I access the array?
I am trying to follow along on Visual Studio so that I have practice in a real world IDE. When you create a react app in Visual Studio an Index.js
file is created that contains the DOM rendering and then an App.js
file is created that contains the top level App
component. I have also created all other components in the App.js
file.
My Index.js
file looks as follows
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { players } from "./App";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App initialPlayers = {players}/>
</React.StrictMode>
);
In the App.js
file, I declared the array at the top like in the tutorial, and then declared the App component as follows:
function App(props) {
return (
<div className="scoreboard">
<Header title="Scoreboard" totalPlayers={1} />{" "}
{props.initialPlayers.map((players) => (
<Player name={props.name} score={props.score} />
))}
</div>
);
}
The IDE is telling me that players
is declared but never used. How is my code unreachable? How would I be able to effectively export the props from the App.js file and reference them from the Index.js file? If this isn't possible or best practice, what do you recommend doing to follow along with this tutorial in Visual Studio. I have tried putting the DOM rendering in the App.js file and deleting Index.js, but I am getting the same warning about my players in the arrow function in the App component being accessed but not declared, so I think there is another issue.
I am not sure what is failing, but when I run the code, despite it compiling, all I render is is a white screen.
Thanks in advance for your help!
1 Answer

Lorenzo Street-Simmons
5,944 PointsIts because you're using props.name and props.score. You need to reference the array using players.name and players.score.
{props.initialPlayers.map( player =>
<Player
name={player.name}
score={player.score}
/>
)}
Seth wright
952 PointsSeth wright
952 PointsThanks for the help Lorenzo. That was definitely a typo and fixed the fact that my players array was declared but never used.
However, the Player and Counter components are not rendering. My App and Header components are rendering, but when I inspect the preview page the Player and Counter components are not there.
My updated
App
component looks as follows:A note on this code block: I was getting an error from the map function, but after Googling, someone else fixed the issue by only mapping if the array existed: hence the ? after the array name
My
Player
component looks like this:The
Counter
component looks like this:My default export at the bottom of the page is App. The
Index.js
file has not changed. Can someone please help explain why my Player and Counter components are not rendering?Thanks!!