Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial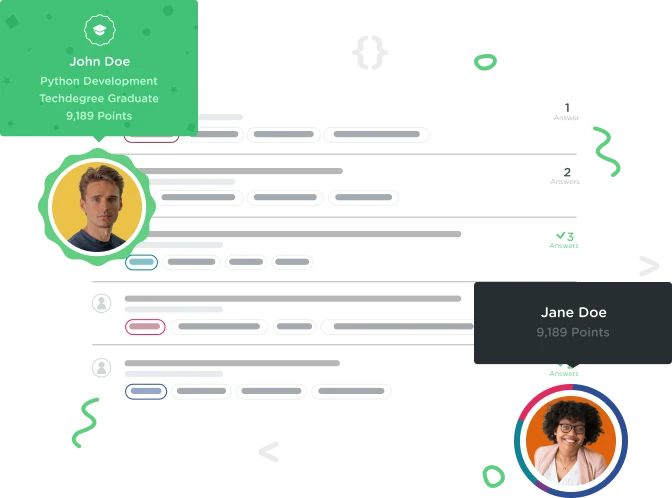

Jeremy Gutzwiller
6,676 PointsDOM Scripting By Example: Last Challenge Question
I am attempting to complete this challenge where I must fill in the proper test condition within the if statement. I have tried to do: if (index.value === law.value). However it is not providing me with the correct output. I know the text value must equal the index value, where am I going wrong?
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index.textContent === law.value) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer
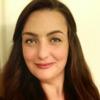
Jennifer Nordell
Treehouse TeacherHi there, Jeremy Gutzwiller ! I received your request for assistance. And you are correct, the value stored in the indexText
(which is storing whatever the user typed in) must equal the index. But remember, we're using the variable i
in our loop to determine which index we're currently looking at.
So as it loops through it should be asking if the index we're looking at currently i
is equal to what is stored in our indexText
variable. Just as an additional note, you will not need any other additional code other than to change what is in those parentheses.
A little pseudocode:
if the `value` stored in `indexText` is equal to the current index we're looking at...
Hope this helps!
edited for better wording

Jeremy Gutzwiller
6,676 PointsThank you for helping me get to the solution!
Takuya Hirata
23,296 PointsTakuya Hirata
23,296 PointsHello Jeremy, your variable "index" contains a number. This means that the index does not have textContent property.
Hope this works/helps.