Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial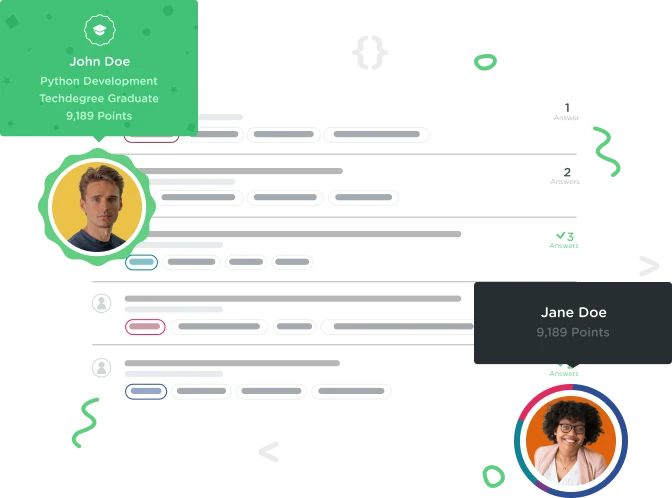

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsDOM Scripting By Example,Implement the makePac() and checkCollisions functions.
let pos = 0; const pacArray = [ ['./images/PacMan1.png', './images/PacMan2.png'], ['./images/PacMan3.png', './images/PacMan4.png'], ]; let direction = 0; const pacMen = []; // This array holds all the pacmen
// This function returns an object with random values function setToRandom(scale) { return { x: Math.random() * scale, y: Math.random() * scale, }; }
// Factory to make a PacMan at a random position with random velocity function makePac() { // returns an object with random values scaled {x: 33, y: 21} let velocity = setToRandom(10); // {x:?, y:?} let position = setToRandom(200);
// Add image to div id = game let game = document.getElementById('game'); let newimg = document.createElement('img'); newimg.style.position = 'absolute'; newimg.src = 'PacMan1.png'; newimg.width = 100;
// TODO: set position here
// TODO add new Child image to game game.appendChild(/* TODO: add parameter */);
// return details in an object return { position, velocity, newimg, }; }
function update() { // loop over pacmen array and move each one and move image in DOM pacMen.forEach((item) => { checkCollisions(item); item.position.x += item.velocity.x; item.position.y += item.velocity.y;
item.newimg.style.left = item.position.x;
item.newimg.style.top = item.position.y;
}); setTimeout(update, 20); }
function checkCollisions(item) { // TODO: detect collision with all walls and make pacman bounce }
function makeOne() { pacMen.push(makePac()); // add a new PacMan }
//don't change this line if (typeof module !== 'undefined') { module.exports = { checkCollisions, update, pacMen }; }
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsAny hints and or suggestions is very much appreciated, thank you in advance for your help.