Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial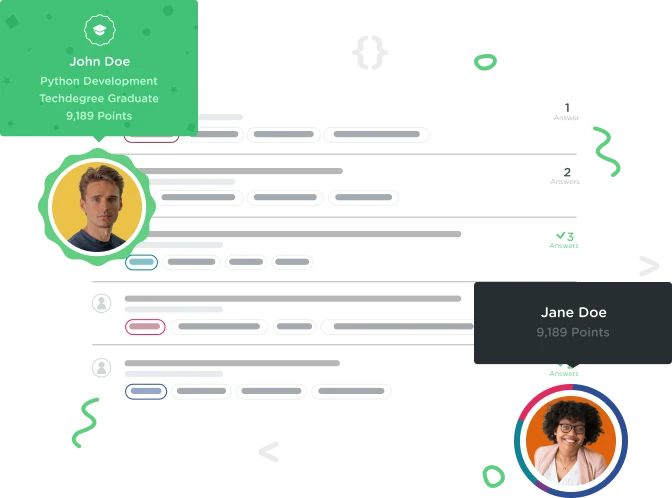

talpaschal
5,616 PointsDOM scripting question midway through "DOM SCRIPTING COURSE"
I know what I'm trying to do I don't understand how my code doesn't work.
I don't even know how to ask for help can someone please look at this and tell me what I'm doing wrong
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index === 0 || index === 1 || index === 2) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer
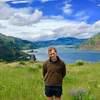
Mckenzie Hessel
Full Stack JavaScript Techdegree Graduate 20,437 PointsSay we enter the number 2 in the text field. With your code as it is now, this is what happens:
- The first time the for loop runs i = 0
- The if condition checks to see if index === 0 || index === 1 || index === 2. Since const index is the number entered in the text field and the user entered the number 2, index === 2. The condition evaluates to true and the next line of code runs
- The next line of code is: law.style.fontWeight = 'bold'. Since const law = laws[i] and i is still equal to 0 at this point, laws[0] (the first list item) is emboldened. We were expecting the third list item to be emboldened, so this is not the desired result
This is what should happen:
- We enter the number 2 in the text field (so const index = 2)
- The for loop runs. It shouldn't embolden laws[0] or laws[1] so it should only evaluate to true on the third time around, when i = 2, to embolden laws[2]. In other words, the code in your if block should only run when a list item's index (i) matches the number entered in the text field (index)

talpaschal
5,616 PointsThanks for responding,
I understand that we want to run the code when what is inputted into the index matches the law[i],
I have tried so many different lines of code I can't get it to work.
whats the point of the code lines including;
const index = parseInt(indexText.value, 10);
&
let law = laws[i];
if (index === law) doesn't work?
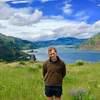
Mckenzie Hessel
Full Stack JavaScript Techdegree Graduate 20,437 PointsThe line const index = parseInt(indexText.value, 10) sets the variable index equal to the number that the user enters in the text field. parseInt just turns the value entered in the text field from a string (for example "0" or "1") into an integer in base 10.
The other line of code (let law = laws[i]) runs each time through the for loop. The first time through, when i = 0, law = laws[0] so law = the first list item. The second time through the loop, when i = 1, law = laws[1]-- the second list item. The third time through, i = 2 so law = laws[2]. Using the if condition (index === law) won't work because the value of index is an integer and the value of law is a list item (either laws[0], laws[1] or laws[2] depending on how many times the for loop has run).
Your if condition should compare const index (the number entered in the text field) to the index of the list item assigned to variable law on each round through the for loop. What variable changes this index each time through the loop? Hint: it's also an integer
talpaschal
5,616 Pointstalpaschal
5,616 Pointsthe conditional statement is wrong and I don't know whats wrong about it