Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial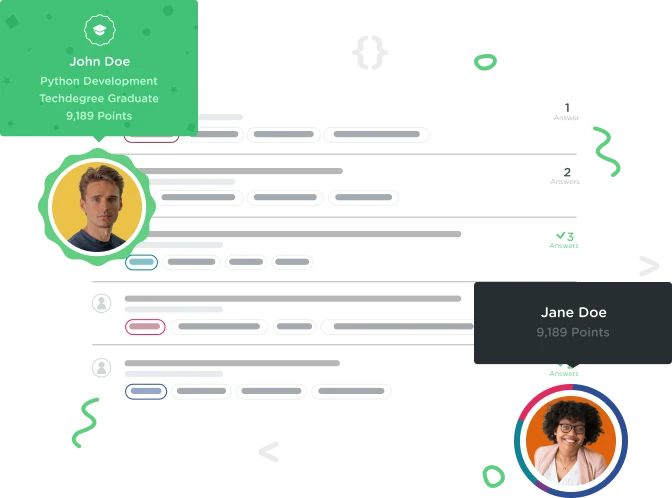
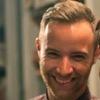
Gibson Smiley
1,923 PointsDone Button
Got lost in task 2 of this challenge.
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem(style: UIBarButton) {
switch style {
case Done(): UIBarButtonStyle.Done
case Edit(): UIBarButtonStyle.Plain
}
}
}
let done = Button.Done("Done")
let doneButon = done
1 Answer
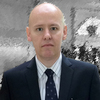
Nathan Tallack
22,160 PointsOk, let me walk you through this one.
In step 2 we are going to create the function within the enum that will be the method we call to turn our done Button instance into a UIBarButtonItem. Consider the following code.
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem { // Here we set return type for the method.
switch self { // Here we switch on self (which is the Button instance we assigned to done.
case .Done(let value): // Here we get that "Done" string and assign it to value.
return UIBarButtonItem(
title: value, // We are using that "Done" string for the title.
style: UIBarButtonStyle.Done,
target: nil,
action: nil)
case .Edit(let value):
return UIBarButtonItem(
title: value,
style: UIBarButtonStyle.Plain,
target: nil,
action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem() // Here we are calling the method on our done Button instance.
The key takeaways here for you are as follows:
- Remember a function inside a type is a method that can be called on itself (in this case the done Button object).
- Remember to use self when referring to the object the method is being called on.
- Remember that associated values for enum cases (like "Done" in this case) can be assigned during the switch (with let value int his case) and used as a variable in the cases of that switch.
I hope this helps. Please do not hesitate to ask any further questions. Everyone on the forum here are happy to help. :)